.NET Core WebApi Interface IP Throttling Practices: Tips for Preventing Malicious Requests
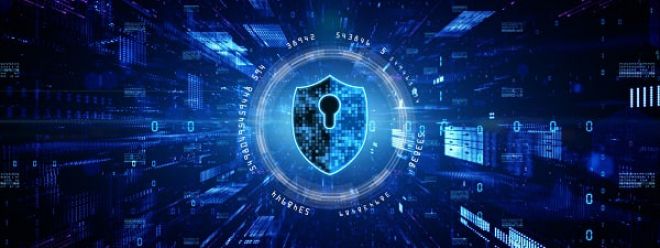
In development. When using the .NET Core WebApi application, we often encounter some uninvited guests - malicious requests or frequent visits. These requests not only increase the burden on the server, but may also affect the access experience of normal users. To combat this, we can protect our WebApi interface by using IP throttling technology, which restricts requests from the same IP address.
1. Understand IP throttling
To put it simply, IP throttling is to control the frequency of requests made by clients based on their IP addresses. If an IP address makes more than a set threshold for a certain period of time, we consider it malicious and restrict it, such as temporarily blocking it for a period of time.
2. Preparations
Before we start implementing IP throttling, we need to do some preparations:
- Specify the throttling policy: You need to set a reasonable throttling policy based on the actual situation of the application. For example, each IP address can be accessed up to 100 times per minute, and the number of visits will be limited beyond that.
- Select the appropriate rate-limiting algorithm: Common rate-limiting algorithms include fixed window counters, sliding window counters, token bucket algorithms, and leaky bucket algorithms. Each algorithm has its own characteristics and application scenarios, and you need to choose according to your actual needs.
- Prepare storage media: In order to record the number of requests per IP, you need a storage medium, such as memory, database, or Redis. The memory is fast, but you will lose data when you restart the app; database persistence, but performance may be limited; Redis is a good compromise that is both fast and long-lasting.
Three. The .NET Core WebAPI implements IP throttling
Next, let's take a look at how to use the . NET Core WebAPI implements IP throttling.
1. Use middleware to throttle
In .NET Core, middleware is a powerful feature that allows us to insert custom code into the pipeline of request processing. We can write a rate-limiting middleware that performs an IP check on each incoming request.
public class IpRateLimitMiddleware
{
private readonly RequestDelegate _next;
private readonly IMemoryCache _memoryCache; // 使用内存缓存来存储IP请求次数
private readonly int _maxRequests; // 最大请求次数
private readonly TimeSpan _timeWindow; // 时间窗口
public IpRateLimitMiddleware(RequestDelegate next, IMemoryCache memoryCache, IOptions<IpRateLimitOptions> options)
{
_next = next;
_memoryCache = memoryCache;
_maxRequests = options.Value.MaxRequests;
_timeWindow = options.Value.TimeWindow;
}
public async Task InvokeAsync(HttpContext context)
{
var clientIp = context.Connection.RemoteIpAddress?.ToString();
if (string.IsNullOrEmpty(clientIp))
{
// 如果无法获取客户端IP,则直接放行
await _next(context);
return;
}
var requestCountKey = $"IpRateLimit:{clientIp}";
if (!_memoryCache.TryGetValue(requestCountKey, out int requestCount))
{
requestCount = 0;
}
// 增加请求次数
requestCount++;
// 检查是否超过限制
if (requestCount > _maxRequests)
{
// 如果超过限制,则根据策略进行处理,比如返回429 Too Many Requests状态码
context.Response.StatusCode = StatusCodes.Status429TooManyRequests;
await context.Response.WriteAsync("Too many requests from this IP address.");
return;
}
// 设置缓存过期时间
_memoryCache.Set(requestCountKey, requestCount, _timeWindow);
// 如果没有超过限制,则继续处理请求
await _next(context);
}
}
// 还需要定义一个配置类IpRateLimitOptions来存储限流策略
public class IpRateLimitOptions
{
public int MaxRequests { get; set; }
public TimeSpan TimeWindow { get; set; }
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
Note: The above code is a simplified example of how to use middleware for IP throttling. In practice, you may need more complex logic to handle different throttling policies, caching mechanisms, error handling, and so on.
2. Register the middleware
Register this middleware in the Startup.cs Configure method:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
// ... 其他中间件配置
app.UseMiddleware<IpRateLimitMiddleware>();
// ... 其他中间件配置
app.UseMvc();
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
Don't forget to inject IMemoryCache and IOptions<IpRateLimitOptions> in the Startup.cs's ConfigureServices method:
public void ConfigureServices(IServiceCollection services)
{
// ... 其他服务配置
services.AddMemoryCache();
services.Configure<IpRateLimitOptions>(Configuration.GetSection("IpRateLimit"));
// ... 其他服务配置
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
And add the appropriate configuration to the appsettings.json:
{
"IpRateLimit": {
"MaxRequests": 100,
"TimeWindow": "00:01:00" // 1分钟时间窗口
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
3. Use a third-party library (e.g. AspNetCoreRateLimit)
Of course, if you find it too cumbersome to implement IP throttling from scratch, you can also consider using an off-the-shelf third-party library, such as AspNetCoreRateLimit. This library provides more powerful and flexible throttling features, including IP throttling, client ID throttling, API endpoint throttling, and more. You can install it via the NuGet package manager and follow the documentation to configure and use it.
4. Precautions
- Performance considerations: When using memory cache for throttling, you need to pay attention to performance issues. If the request volume is very high, the memory consumption can be high. At this point, you can consider using a distributed cache such as Redis to optimize performance.
- Error handling: During throttling, you may encounter various errors, such as cache access failures and configuration read errors. You need to handle errors well, make sure you can give clear warnings when something goes wrong, and take action accordingly.
- Logging: To facilitate debugging and monitoring, we recommend that you add a logging feature to record information such as the IP address, request time, and throttling policy during the throttling process.
- Policy adjustment: The throttling policy is not static, and you need to continuously adjust and optimize the throttling policy based on the actual situation of the app and user feedback.
5. Summary
Through the above introduction, we have learned how to use . NET Core WebAPI implements IP throttling. From understanding IP throttling, to preparation, to middleware implementation and precautions, each step is explained in detail. Hopefully, this article will help you better understand and implement this feature to protect your WebApi interface from malicious requests.