Network analysis in Rust: Capture and analyze network traffic using Pcap and Pnet
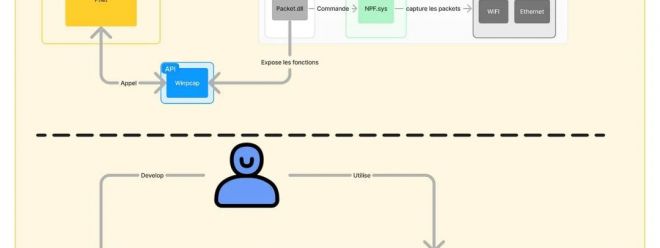
Network analytics is essential for monitoring, securing, and diagnosing network infrastructure, and Rust is becoming an increasingly popular choice for developers building networking tools due to its performance and memory safety.
In this article, we'll explore how to use pcap and pnet in Rust to read PCAP files, capture real-time network traffic, and briefly discuss high-performance packet capture with PF_RING.
Use pcap to read the PCAP file
The pcap library allows you to read files captured from the network, often referred to as PCAP (Packet Capture), which contain traces of network traffic, a step that is essential for analyzing network events or debugging.
A simple example of reading a packet from a file:
use pcap::Capture;
fn main() {
let mut cap = Capture::from_file("example.pcap").unwrap();
while let Ok(packet) = cap.next() {
println!("Packet : {:?}", packet);
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
Use PNET for fine-grained packet capture and analysis
pnet crate allows the use of lower-level network packets in Rust. Unlike pcap, it provides a more detailed API to manipulate packet headers, protocols, and access NICs through system libraries.
Pnet embeds the original socket of the operating system into the crate:
use pnet::datalink::{self, Channel::Ethernet};
fn main() {
let interfaces = datalink::interfaces();
let interface = interfaces.into_iter()
.find(|iface| iface.is_up() && !iface.is_loopback())
.expect("No suitable interface found.");
let (_, mut rx) = match datalink::channel(&interface, Default::default()) {
Ok(Ethernet(tx, rx)) => (tx, rx),
Ok(_) => panic!("Unhandled channel type."),
Err(e) => panic!("An error occurred: {}", e),
};
loop {
match rx.next() {
Ok(packet) => println!("Packet : {:?}", packet),
Err(e) => eprintln!("An error occurred while reading: {}", e),
}
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
Use PNET and LIBC to access the NIC
In order to efficiently capture and filter packets, PNET can interact directly with the system library. On Windows, this is done through Npcap (a fork of WinPcap) and on Linux with raw sockets and Berkeley Packet Filters (BPF). libc is typically used to access these system-level features.
Image
PNET uses system calls to access network drivers through libraries such as libc.
For environments that require high performance, you can use PF_RING to optimize capture by accessing the NIC directly.
summary
Rust provides a variety of powerful tools for network analysis and capture, and pcap and pnet provide features suitable for different levels of abstraction. For the capture and detailed analysis of network data, as well as the need for high performance, PNET and PF_RING are particularly suitable.