Vue 3 advanced responsive data exploration: principles, usage details and practical examples!
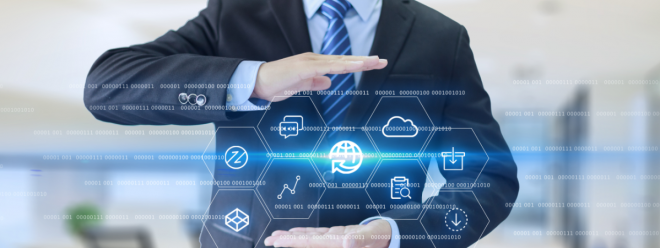
In Vue 3, data changes are implemented through a reactive system based on the ES6 Proxy object. Proxy objects allow interception and custom operations, so Vue can monitor data and trigger corresponding operations through proxy objects. The following is a detailed description of the principle, usage and steps of monitoring data changes in Vue 3, as well as an example code:
principle:
The responsive system of Vue 3 is based on the Proxy object, which intercepts data through the proxy object to monitor data changes. When data is accessed or modified, Proxy will trigger corresponding operations, such as updating views.
Instructions:
- reactive function: Use the reactive function to create a reactive object.
- ref function: Use the ref function to create a reactive object containing a value property, suitable for basic data types.
- toRefs function: Converts a reactive object into a reactive reference of a normal object.
- watch function : monitor changes in data and execute a custom callback function when the data changes.
step:
Step 1: Install Vue 3
npm install vue@next
- 1.
Step 2: Create a Vue instance and use reactive data
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vue 3高级响应式数据</title>
</head>
<body>
<div id="app">
<p>{{ user.name }}</p>
<p>{{ user.age }}</p>
<button @click="updateUser">更新用户</button>
</div>
<script src="https://unpkg.com/vue@next"></script>
<script src="main.js"></script>
</body>
</html>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
Step 3: Write JavaScript code for Vue instances and reactive data
// main.js
import { createApp, reactive, toRefs, watch } from 'vue';
// 创建Vue实例
const app = createApp({
// 使用响应式数据
setup() {
// 创建响应式对象
const user = reactive({
name: 'John',
age: 25
});
// 监听响应式数据的变化
watch(() => {
console.log('用户信息发生变化:', user.name, user.age);
});
// 定义更新用户的方法
const updateUser = () => {
// 修改响应式数据
user.name = 'Jane';
user.age += 1;
};
// 返回响应式对象的引用
return {
user: toRefs(user),
updateUser
};
}
});
// 挂载Vue实例到HTML元素上
app.mount('#app');
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
In the above advanced example, we used the reactive function to create a reactive object user containing name and age properties . Through the toRefs function, we convert the reactive object into a reactive reference of a normal object, so that user.name and user.age can be used directly in the template . At the same time, we use the watch function to monitor changes in the user object. When the data changes, information will be output to the console.