Spring Boot email sending tutorial: step by step, easily implement image attachment email!
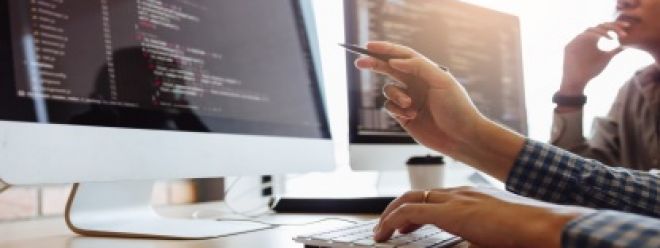
Step 1: Add dependencies
Make sure to add the following dependencies in your pom.xml file to introduce Spring Boot’s email support:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
- 1.
- 2.
- 3.
- 4.
Step 2: Create mail service class
Create a service class that contains the logic for sending emails with image attachments. In this example, we use JavaMailSender and MimeMessageHelper to build the mail:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.ByteArrayResource;
import org.springframework.core.io.Resource;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
@Service
public class EmailService {
@Autowired
private JavaMailSender javaMailSender;
public void sendEmailWithAttachment(String to, String subject, String text, String imagePath) throws MessagingException, IOException {
MimeMessage message = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(text, true);
// 添加图片附件
helper.addInline("imageAttachment", getImageResource(imagePath));
javaMailSender.send(message);
}
private Resource getImageResource(String imagePath) throws IOException {
Path path = Paths.get(imagePath);
byte[] imageBytes = Files.readAllBytes(path);
return new ByteArrayResource(imageBytes);
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
Step 3: Create a Controller for sending emails
Create a Controller class to trigger the action of sending emails with image attachments:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.mail.MessagingException;
import java.io.IOException;
@RestController
@RequestMapping("/email")
public class EmailController {
@Autowired
private EmailService emailService;
@GetMapping("/send")
public String sendEmailWithAttachment() {
try {
// 替换为实际的收件人地址、主题、邮件内容和图片路径
String to = "recipient@example.com";
String subject = "邮件主题";
String text = "邮件正文,包含图片:<img src='cid:imageAttachment'/>"; // 注意使用cid:imageAttachment引用图片附件
String imagePath = "/path/to/your/image.jpg";
emailService.sendEmailWithAttachment(to, subject, text, imagePath);
return "邮件发送成功";
} catch (MessagingException | IOException e) {
e.printStackTrace();
return "邮件发送失败";
}
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
Step 4: Run the application
Make sure the Spring Boot application is configured correctly and run the application. By accessing the defined Controller interface, trigger the operation of sending emails with image attachments.
The code in this example is a basic implementation and you may need to make appropriate modifications and extensions based on actual needs. Make sure to replace the placeholders in the examples (such as recipient addresses, subject, message content, and image paths) with actual values.