ABP framework for beginners and precautions: pure backend perspective
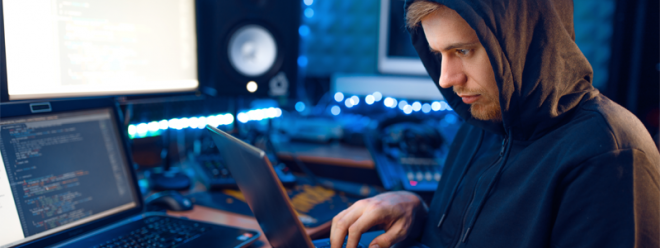
The ABP (ASP.NET Boilerplate) framework is an open source framework for building modular, multi-tenant applications. It provides a complete set of development infrastructure, including many best practices of domain-driven design (DDD), modular design, multi-tenant support, authentication and authorization, exception handling, logging, etc. For novices, the ABP framework can greatly speed up the development process, but at the same time, you also need to pay attention to some key issues to ensure the smooth progress of the project.
1. Introduction to ABP Framework
The ABP framework is based on .NET Core and Entity Framework Core. It follows the principles of domain-driven design (DDD) and provides rich features to help developers quickly build enterprise-level applications. By using the ABP framework, developers can focus more on the implementation of business logic without having to worry too much about the underlying technical details.
2. Notes for novices using the ABP framework
- Learn Domain Driven Design (DDD): ABP framework is built based on DDD, so understanding the basic concepts of DDD like Aggregate, Entity, Value Object, Domain Service, etc. is crucial to use ABP effectively.
- Modular Design: ABP supports modular development, each module has its own functions and services. Newbies should make full use of this feature and split the application into multiple modules to improve the maintainability and scalability of the code.
- Exception handling and logging: ABP provides a powerful exception handling and logging mechanism. Make sure to properly handle exceptions in your code and record necessary log information for easy debugging and troubleshooting.
- Authentication and Authorization: ABP integrates authentication and authorization mechanisms. Proper configuration and use of these features can ensure the security of your application.
- Performance optimization: Although the ABP framework itself has performed a lot of performance optimization, in actual development, you still need to pay attention to avoiding N+1 query problems, using cache properly, and other performance-related best practices.
3. Sample Code
The following is a simple example of using ABP Framework, showing how to create a simple domain entity and service.
1. Define domain entities
First, we define a simple Product entity:
using Abp.Domain.Entities;
using Abp.Domain.Entities.Auditing;
public class Product : Entity<long>, IHasCreationTime
{
public string Name { get; set; }
public decimal Price { get; set; }
public DateTime CreationTime { get; set; }
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
2. Create domain services
Next, we create a simple domain service to handle the business logic of the Product entity:
using Abp.Domain.Services;
using System.Collections.Generic;
using System.Linq;
public class ProductManager : DomainService
{
private readonly IRepository<Product, long> _productRepository;
public ProductManager(IRepository<Product, long> productRepository)
{
_productRepository = productRepository;
}
public virtual void CreateProduct(string name, decimal price)
{
var product = new Product
{
Name = name,
Price = price,
CreationTime = Clock.Now // 使用ABP提供的Clock服务获取当前时间
};
_productRepository.Insert(product);
}
public virtual List<Product> GetAllProducts()
{
return _productRepository.GetAllList();
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
3. Use Domain Services
In the application service layer, you can call ProductManager to handle business logic:
public class ProductAppService : ApplicationService, IProductAppService
{
private readonly ProductManager _productManager;
public ProductAppService(ProductManager productManager)
{
_productManager = productManager;
}
public void Create(CreateProductInput input)
{
_productManager.CreateProduct(input.Name, input.Price);
}
public List<ProductDto> GetAll()
{
var products = _productManager.GetAllProducts();
return ObjectMapper.Map<List<ProductDto>>(products); // 使用ABP的ObjectMapper进行DTO映射
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
In this example, we showed how to define domain entities, create domain services, and use these services in the application service layer in the ABP Framework. Please note that to simplify the example, we omitted some features and best practices of the ABP Framework, such as dependency injection, validation, permission checking, etc. In a real project, you should improve these aspects according to your specific needs.
IV. Conclusion
The ABP framework provides developers with a powerful infrastructure to build modular and scalable applications. As a novice, mastering the basic principles of DDD, modular design, exception handling and logging and other key concepts is essential for the successful use of ABP. Through continuous learning and practice, you will be able to fully utilize the advantages of the ABP framework and quickly build high-quality enterprise-level applications.