Commonly used gadgets in the interface automation framework
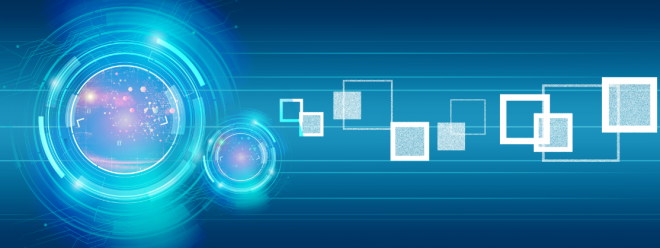
In daily programming work, we often need to deal with various issues related to time, data format and configuration files. This article has compiled a series of practical Python code snippets, covering date and time conversion, data formatting and conversion, obtaining file comments, reading configuration files, etc., to help developers improve work efficiency and easily handle common tasks.
1. Second-level and millisecond-level timestamp acquisition
# 获取当前秒级时间戳
def millisecond(add=0):
return int(time.time()) + add
# 获取当前毫秒级时间戳
def millisecond_new():
t = time.time()
return int(round(t * 1000))
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
These two functions provide the function of obtaining the second-level and millisecond-level timestamp of the current time respectively. The millisecond() function allows passing in an optional parameter add, which is used to increase the specified time offset.
2. Get the current date string
#获取当前时间日期: 20211009
def getNowTime(tianshu=0):
shijian = int(time.strftime('%Y%m%d')) - tianshu
print(shijian)
return shijian
- 1.
- 2.
- 3.
- 4.
- 5.
The getNowTime() function returns the current date (in the format YYYYMMDD) and supports passing in the parameter tianshu to subtract the specified number of days. This function is suitable for situations where you need to process date data and only focus on the year, month and day.
3. Repair the interface to return unquoted JSON data
def json_json():
with open("源文件地址", "r") as f, open("目标文件地址", "a+") as a:
a.write("{")
for line in f.readlines():
if "[" in line.strip() or "{" in line.strip():
formatted_line = "'" + line.strip().replace(":", "':").replace(" ", "") + ","
print(formatted_line) # 输出修复后的行
a.write(formatted_line + "\n")
else:
formatted_line = "'" + line.strip().replace(":", "':'").replace(" ", "") + "',"
print(formatted_line) # 输出修复后的行
a.write(formatted_line + "\n")
a.write("}")
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
This function is used to process improperly formatted JSON data copied from the interface, fix the missing quotes, and write it to a new file. The paths of source and target files need to be replaced with actual paths.
4. Convert URL query string to JSON
from urllib.parse import urlsplit, parse_qs
def query_json(url):
query = urlsplit(url).query
params = dict(parse_qs(query))
cleaned_params = {k: v[0] for k, v in params.items()}
return cleaned_params
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
The query_json() function receives a URL containing a query string, parses its query part, converts it to dictionary form, and cleans multi-valued parameters so that only the first value is retained.
5. File comment extraction
import os
def get_first_line_comments(directory, output_file):
python_files = sorted([f for f in os.listdir(directory) if f.endswith('.py') and f != '__init__.py'])
comments_and_files = []
for file in python_files:
filepath = os.path.join(directory, file)
with open(filepath, 'r', encoding='utf-8') as f:
first_line = f.readline().strip()
if first_line.startswith('#'):
comment = first_line[1:].strip()
comments_and_files.append((file, comment))
with open(output_file, 'w', encoding='utf-8') as out:
for filename, comment in comments_and_files:
out.write(f"{filename}: {comment}\n")
# 示例用法
get_first_line_comments('指定文件夹', '指定生成文件路径.txt')
get_first_line_comments()函数遍历指定目录下的.py文件,提取每份文件的第
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
6. Read the configuration INI file
import sys
import os
import configparser
class ReadConfig:
def __init__(self, config_path):
self.path = config_path
def read_sqlConfig(self, fileName="sql.ini"):
read_mysqlExecuteCon = configparser.ConfigParser()
read_mysqlExecuteCon.read(os.path.join(self.path, fileName), encoding="utf-8")
return read_mysqlExecuteCon._sections
def read_hostsConfig(self, fileName="hosts.ini"):
read_hostsCon = configparser.ConfigParser()
read_hostsCon.read(os.path.join(self.path, fileName), encoding="utf-8")
return read_hostsCon._sections
# 示例用法
config_reader = ReadConfig('配置文件所在路径')
sql_config = config_reader.read_sqlConfig()
hosts_config = config_reader.read_hostsConfig()["hosts"]
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
7.Set global file path
import os
def setFilePath(filePath):
current_module_path = os.path.dirname(os.path.abspath(__file__))
project_root_path = os.path.dirname(os.path.dirname(current_module_path))
path = os.path.join(project_root_path, filePath.lstrip('/'))
return os.path.abspath(path)
# 示例用法
confPath = setFilePath("地址文件路径")
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
The setFilePath() function calculates the absolute path of the target file or directory in the project root directory based on the provided relative path and the absolute path of the current module, which facilitates unified management of resource locations in the project.