A two-way communication without confirmation IP through Udp
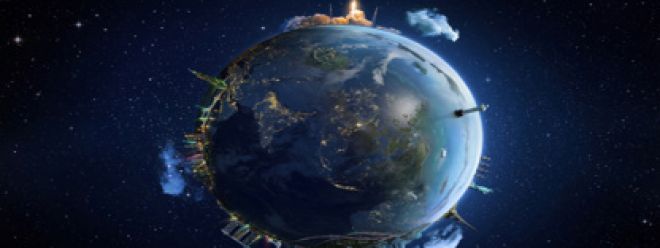
A two-way communication without confirmation IP through Udp
foreword
UDP is an unreliable communication, but it is still used sometimes. Share an example today: the main logic, a port broadcast address, after receiving the ip address data, other ports are bound based on this ip, and finally communicate, so that we can ensure that we do not need to pay attention to ip address binding when we continue to increase port interaction in the future The problem.
Introduction to the main principles
- The low communication frequency port performs udp broadcast of server IP information, and the receiving end is not fixed IP monitoring, listening to a specific port of any IP address of the host
- After receiving the ip address of the broadcast channel, establish tcp or udp two-way high-frequency communication with the specific IP and port.
The figure below is the calling process of the UDP-based Socket function:
Bind ip and port are only required when receiving
socket listens on all ip specific port codes:
#define PORT 6000
bzero(&adr_inet, sizeof(adr_inet));
adr_inet.sin_family = AF_INET;
adr_inet.sin_addr.s_addr = htonl(INADDR_ANY);
adr_inet.sin_port = htons(port);
ret = bind(cfd, (struct sockaddr *)&addr, sizeof(addr));
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
The description of the ip bound to the socket as INADDR_ANY:
socket INADDR_ANY listens to the address 0.0.0.0, the socket only binds the port and lets the routing table decide which ip to send to
Among them, INADDR_ANY is the address whose specified address is 0.0.0.0. This address actually represents an uncertain address, or "all addresses" or "any address". If the ip address is specified as a wildcard address (INADDR_ANY), then the kernel will wait until the socket is connected (TCP) or a datagram has been sent on the socket before choosing a local IP address. In general, if you want to build a network server, you need to notify the server operating system: Please listen on a certain port yyyy on a certain address xxx.xxx.xxx.xxx, and send the intercepted data packets to I. You do this through the bind() system call. ——That is to say, your program needs to bind a certain address of the server, or say: take a certain port on a certain address of the server as used. The server operating system may or may not give you this specified address.
If your server has multiple network cards, and your service (whether listening on udp port, or listening on tcp port), for some reason: it may be that your server operating system may increase or decrease IP at any time The address may also be used to save the trouble of determining what network port (network card) is on the server-you can tell the operating system when calling bind(): "I need to listen on port yyyy, so send it to the server No matter which network card/IP address receives the data, it is all processed by me." At this time, the server is listening on the address 0.0.0.0. No matter which ip is connected, it can be connected, as long as all ips sent to this port can be connected.
Sample code:
data_send.c performs udp broadcast of ip address on port 9001 and broadcasts read terminal data to port 7000
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <errno.h>
#include <pthread.h>
#include <signal.h>
#define IP "127.0.0.1"
#define
#define
// gcc data_send.c -o data_send -pthread
int cfd = -1;
//接收线程函数
void *receive(void *pth_arg)
{
int ret = 0;
char name_data[3] = {0};
struct sockaddr_in addr0 = {0};
int addr0_size = sizeof(addr0);
//从对端ip和端口号中接收消息,指定addr0用于存放消息
while (1)
{
bzero(name_data, sizeof(name_data));
ret = recvfrom(cfd, name_data, sizeof(name_data), 0, (struct sockaddr *)&addr0, &addr0_size);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "recv failed", strerror(errno));
exit(-1);
}
else if (ret > 0)
{
printf("\nname = %s ", name_data); //打印对方的消息和端口号
printf("ip %s,port %d \n", inet_ntoa(addr0.sin_addr), ntohs(addr0.sin_port));
}
}
}
void *data_send(void *pth_arg)
{
int ret = 0;
char data[] = "IP address";
struct sockaddr_in addr0 = {0};
addr0.sin_family = AF_INET; //设置tcp协议族
addr0.sin_port = htons(DATA_PORT); //设置端口号
addr0.sin_addr.s_addr = htonl(INADDR_ANY); //设置ip地址
//发送消息
while (1)
{
ret = sendto(cfd, (void *)data, sizeof(data), 0, (struct sockaddr *)&addr0, sizeof(addr0));
sleep(1);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "sendto failed", strerror(errno));
exit(-1);
}
}
}
int main()
{
int ret = -1;
//创建tcp/ip协议族,指定通信方式为无链接不可靠的通信
cfd = socket(AF_INET, SOCK_DGRAM, 0);
if (-1 == cfd)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "socket failed", strerror(errno));
exit(-1);
}
//进行端口号和ip的绑定
struct sockaddr_in addr;
addr.sin_family = AF_INET; //设置tcp协议族
addr.sin_port = htons(PORT); //设置端口号
addr.sin_addr.s_addr = inet_addr(IP); //设置ip地址
ret = bind(cfd, (struct sockaddr *)&addr, sizeof(addr));
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "bind failed", strerror(errno));
exit(-1);
}
//创建线程函数,用于处理数据接收
pthread_t id,data_send_id;
ret = pthread_create(&id, NULL, receive, NULL);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "pthread_create failed", strerror(errno));
exit(-1);
}
// pthread_join(id,NULL);
ret = pthread_create(&data_send_id, NULL, data_send, NULL);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "pthread_create failed", strerror(errno));
exit(-1);
}
struct sockaddr_in addr0;
addr0.sin_family = AF_INET; //设置tcp协议族
addr0.sin_port = htons(7000); //设置端口号
addr0.sin_addr.s_addr = inet_addr(IP); //设置ip地址
char name_send[3] = {0};
//发送消息
while (1)
{
bzero(name_send, sizeof(name_send));
printf("send name:");
scanf("%s", name_send);
//发送消息时需要绑定对方的ip和端口号
ret = sendto(cfd, (void *)name_send, sizeof(name_send), 0, (struct sockaddr *)&addr0, sizeof(addr0));
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "accept failed", strerror(errno));
exit(-1);
}
}
return 0;
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
- 63.
- 64.
- 65.
- 66.
- 67.
- 68.
- 69.
- 70.
- 71.
- 72.
- 73.
- 74.
- 75.
- 76.
- 77.
- 78.
- 79.
- 80.
- 81.
- 82.
- 83.
- 84.
- 85.
- 86.
- 87.
- 88.
- 89.
- 90.
- 91.
- 92.
- 93.
- 94.
- 95.
- 96.
- 97.
- 98.
- 99.
- 100.
- 101.
- 102.
- 103.
- 104.
- 105.
- 106.
- 107.
- 108.
- 109.
- 110.
- 111.
- 112.
- 113.
- 114.
- 115.
- 116.
- 117.
- 118.
- 119.
- 120.
- 121.
- 122.
- 123.
- 124.
- 125.
- 126.
- 127.
data_process.c captures the ip data of port 9001. When the ip data is received, it binds the broadcast ip address to send and receive data. Here, udp is used for receiving. You can also try tcp interaction.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <errno.h>
#include <pthread.h>
#include <signal.h>
#define IP "127.0.0.1"
#define
#define
// typedef uint32_t in_addr_t;
// gcc data_process.c -o data_process -pthread
int cfd = -1,data_fd = -1;
uint32_t receive_ip = -1;
void *receive(void *pth_arg)
{
int ret = 0;
char name_data[3] = {0};
struct sockaddr_in addr0 = {0};
int addr0_size = sizeof(addr0);
while (1)
{
printf("receive:");
bzero(name_data, sizeof(name_data));
ret = recvfrom(cfd, name_data, sizeof(name_data), 0, (struct sockaddr *)&addr0, &addr0_size);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "recv failed", strerror(errno));
exit(-1);
}
else if (ret > 0)
{
printf("\nname = %s ", name_data);
printf("ip %s,port %d \n", inet_ntoa(addr0.sin_addr), ntohs(addr0.sin_port));
}
}
}
void *data_receive(void *pth_arg)
{
int ret = 0;
char name_data[10] = {0};
struct sockaddr_in addr0 = {0};
int addr0_size = sizeof(addr0);
while (1)
{
bzero(name_data, sizeof(name_data));
ret = recvfrom(data_fd, name_data, sizeof(name_data), 0, (struct sockaddr *)&addr0, &addr0_size);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "recv failed", strerror(errno));
exit(-1);
}
else if (ret > 0)
{
printf("\nname = %s ", name_data);
printf("ip %s,port %d \n", inet_ntoa(addr0.sin_addr), ntohs(addr0.sin_port));
receive_ip = addr0.sin_addr.s_addr;
char buf[20] = { 0 };
inet_ntop(AF_INET, &receive_ip, buf, sizeof(buf));
printf("receive_ip ip = %s ", buf);
// printf("receive_ip ip = %s ", inet_ntop(receive_ip));
break;
}
}
}
int main()
{
int ret = -1;
data_fd = socket(AF_INET, SOCK_DGRAM, 0);
if (-1 == data_fd)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "socket failed", strerror(errno));
exit(-1);
}
struct sockaddr_in addr;
addr.sin_family = AF_INET; //设置tcp协议族
addr.sin_port = htons(DATA_PORT); //设置端口号
addr.sin_addr.s_addr = inet_addr(IP); //设置ip地址
ret = bind(data_fd, (struct sockaddr *)&addr, sizeof(addr));
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "bind failed", strerror(errno));
exit(-1);
}
pthread_t receive_id;
ret = pthread_create(&receive_id, NULL, data_receive, NULL);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "pthread_create failed", strerror(errno));
exit(-1);
}
pthread_join(receive_id,NULL);
cfd = socket(AF_INET, SOCK_DGRAM, 0);
if (-1 == cfd)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "socket failed", strerror(errno));
exit(-1);
}
struct sockaddr_in addr1;
addr1.sin_family = AF_INET; //设置tcp协议族
addr1.sin_port = htons(PORT); //设置端口号
addr1.sin_addr.s_addr = receive_ip; //设置ip地址
char buf[20] = { 0 };
inet_ntop(AF_INET, &receive_ip, buf, sizeof(buf));
printf("ip = %s ", buf);
ret = bind(cfd, (struct sockaddr *)&addr1, sizeof(addr1));
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "bind failed", strerror(errno));
exit(-1);
}
pthread_t id;
ret = pthread_create(&id, NULL, receive, NULL);
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "pthread_create failed", strerror(errno));
exit(-1);
}
pthread_join(id,NULL);
struct sockaddr_in addr0;
addr0.sin_family = AF_INET; //设置tcp协议族
addr0.sin_port = htons(6000); //设置端口号
addr0.sin_addr.s_addr = inet_addr(IP); //设置ip地址
char name_send[3] = {0};
while (1)
{
bzero(name_send, sizeof(name_send));
printf("send name:");
scanf("%s", name_send);
ret = sendto(cfd, (void *)name_send, sizeof(name_send), 0, (struct sockaddr *)&addr0, sizeof(addr0));
if (-1 == ret)
{
fprintf(stderr, "%d, %s :%s", __LINE__, "accept failed", strerror(errno));
exit(-1);
}
}
return 0;
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
- 63.
- 64.
- 65.
- 66.
- 67.
- 68.
- 69.
- 70.
- 71.
- 72.
- 73.
- 74.
- 75.
- 76.
- 77.
- 78.
- 79.
- 80.
- 81.
- 82.
- 83.
- 84.
- 85.
- 86.
- 87.
- 88.
- 89.
- 90.
- 91.
- 92.
- 93.
- 94.
- 95.
- 96.
- 97.
- 98.
- 99.
- 100.
- 101.
- 102.
- 103.
- 104.
- 105.
- 106.
- 107.
- 108.
- 109.
- 110.
- 111.
- 112.
- 113.
- 114.
- 115.
- 116.
- 117.
- 118.
- 119.
- 120.
- 121.
- 122.
- 123.
- 124.
- 125.
- 126.
- 127.
- 128.
- 129.
- 130.
- 131.
- 132.
- 133.
- 134.
- 135.
- 136.
- 137.
- 138.
- 139.
- 140.
- 141.
- 142.
- 143.
- 144.
- 145.
- 146.
- 147.
- 148.
- 149.
- 150.
- 151.
- 152.
One terminal captures data, sudo tcpdump -i lo portrange 5000-8000 -vv -XX -nn, and the other two terminals exchange data
epilogue
This is the sharing of some of my own udp design ideas. If you have better ideas and needs, you are also welcome to add my friends to exchange and share.
Author: I still have a conscience, work hard during the day, and create an official account owner at night. In addition to technology, the content of the official account also has some life insights. A veteran driver in the workplace who is serious about outputting content is also a person who enriches life other than technology, photography, music and basketball.