TCP Service Construction in Go: Principles to Engineering Practices
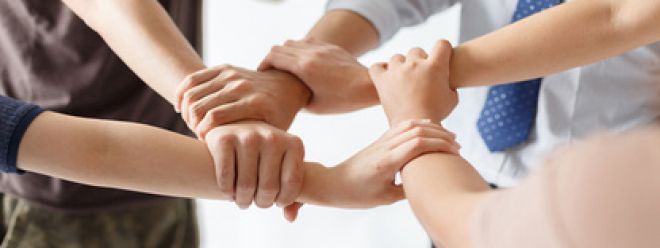
Transport layer protocols play a key role in distributed system architectures. As a representative of reliable transmission, the TCP protocol provides an orderly and reliable data transmission channel for upper-layer applications through technologies such as three-way handshake connection, sliding window flow control, and serial number confirmation mechanism. This connection-oriented protocol makes it the preferred solution for real-time communication, file transfer, remote control, and more.
Since its inception, Go has had network programming capabilities at its core. The complete .net package in the standard library provides a cross-platform network I/O interface, combined with lightweight thread goroutines and efficient schedulers, enabling developers to build high-performance network services with simple code. This native support at the language level significantly lowers the threshold for concurrent server development.
Analysis of the basic service architecture
Network layer initialization process
The starting point for creating a TCP service is port listening. In Go, net. The listen("tcp", address) function accomplishes several important operations:
1. Parse the address format and separate the IP address and port
2. Create a socket file descriptor
3. Bind the specified port
4. Enter the listening state
The Listener object returned by this function maintains the connection queue on the server side, and implements the connection management mechanism at the operating system level. Developers don't need to worry about the details of the underlying socket to get a usable listening interface.
The lifecycle of the connection process
The typical process for a server to receive a connection consists of three key stages:
1. The Accept() method blocks and waits for the client to connect
2. Get the net that represents the connection. Conn object
3. Start a stand-alone processing coroutine
This pattern ensures that the server can handle multiple client requests at the same time. Each Conn object encapsulates local and remote address information, as well as the underlying data transmission channel.
An example of a simplified service implementation
package main
import (
"log"
"net"
)
func handleConnection(conn net.Conn) {
defer conn.Close()
buffer := make([]byte, 1024)
for {
n, err := conn.Read(buffer)
if err != nil {
log.Println("读取错误:", err)
return
}
message := string(buffer[:n])
log.Printf("收到 %s: %s", conn.RemoteAddr(), message)
if _, err := conn.Write([]byte("已接收\n")); err != nil {
log.Println("写入错误:", err)
return
}
}
}
func main() {
listener, err := net.Listen("tcp", ":8080")
if err != nil {
log.Fatal("监听失败:", err)
}
defer listener.Close()
log.Println("服务启动,监听端口 8080")
for {
conn, err := listener.Accept()
if err != nil {
log.Println("接受连接失败:", err)
continue
}
go handleConnection(conn)
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
Analysis of key technical points
Concurrency processing mechanism
The use of go handleConnection(conn) in this example exemplifies Go's philosophy of concurrency. Each connection is handled in a separate goroutine, and these lightweight threads are scheduled by the Go runtime, multiplexing on top of the operating system threads. Compared with traditional thread pool schemes, this model significantly reduces memory consumption and context switching costs.
Data buffer management
A buffer of 1024 bytes is a typical choice for trade-offs between memory usage and processing efficiency. In practice, the following needs to be considered:
1. The maximum packet length of the application protocol
2. Memory usage efficiency
3. Optimized the number of system calls
For streaming scenarios, packet framing logic at the application layer needs to be implemented, including length prefix method or delimiter detection.
Error Handling Policies
Error handling in network programming needs to distinguish between temporary and fatal errors:
• Transient errors, such as transient network outages, often require a retry mechanism
• A protocol error requires the current connection to be interrupted
• System-level errors, such as running out of file descriptors, may require a service restart
In this example, the error handling method is hierarchically recorded, and the actual production environment needs to be combined with the monitoring system to classify alarms.
Production Environment Enhancements
Connection control parameters
Via net. TCPListener's type assertion can set the underlying socket parameters:
if tcpListener, ok := listener.(*net.TCPListener); ok {
tcpListener.SetKeepAlive(true)
tcpListener.SetKeepAlivePeriod(3 * time.Minute)
}
- 1.
- 2.
- 3.
- 4.
This type of parameter optimization needs to be adjusted according to the actual network environment, such as NAT timeout period and carrier policies.
Graceful termination implementation
Add signal monitoring to achieve safe shutdown:
sigCh := make(chan os.Signal, 1)
signal.Notify(sigCh, syscall.SIGINT, syscall.SIGTERM)
go func() {
<-sigCh
listener.Close()
}()
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
This solution ensures that the service is able to complete the request being processed and avoids data loss.
Performance optimization direction
1. Use sync. Pool reuses buffer objects
2. Limit the maximum number of concurrent connections
3. Achieve zero-copy data transfer
4. Reduce memory allocation with ring buffers
Typical application scenario expansion
Protocol Design Practices
Build an application-layer protocol on top of the basic example:
type Message struct {
Header uint16
Length uint32
Payload []byte
CRC uint32
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
This structured protocol design supports functions such as message routing, integrity checking, and more.
Secure transport schemes
Enhance transmission security with TLS encryption:
cert, _ := tls.LoadX509KeyPair("server.pem", "server.key")
config := &tls.Config{Certificates: []tls.Certificate{cert}}
listener, err = tls.Listen("tcp", ":443", config)
- 1.
- 2.
- 3.
In this way, the transmission is encrypted while maintaining the consistency of the interface.
Architecture Evolution Roadmap
The evolution from stand-alone services to distributed systems needs to consider:
1. Load balancing strategy
2. Service discovery mechanism
3. Synchronization of connection status
4. Distributed tracing integration
Modern cloud-native architectures typically integrate TCP services with infrastructure such as Service Mesh to implement advanced features such as traffic management and observability.
Advice on engineering practices
1. Use PPROF for performance analysis
2. Integrate Prometheus monitoring metrics
3. Implement a connected heartbeat mechanism
4. Design a stress test plan
5. Establish an exception recovery strategy
In the microservice architecture, TCP services often exist as sidecar proxies or dedicated gateways, and special attention needs to be paid to resource constraints and the implementation of circuit breaker mechanisms.
Through continuous optimization and iteration, the TCP service built on Go can support millions of concurrent connections and show excellent performance in instant messaging, Internet of Things, financial transactions, and other fields. This evolutionary process from simple to complex is the essence of engineering practice.