Spring's elegant flow of state data
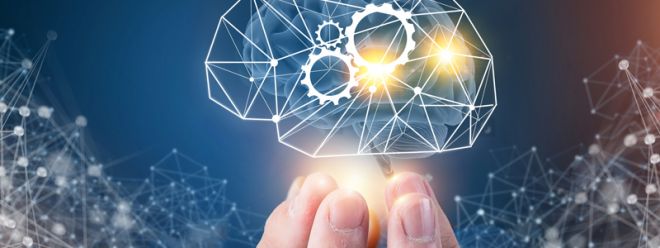
When using Spring Statemachine (state machine), you can follow the following steps to use and implement:
- Introduce dependencies: Introduce Spring Statemachine dependencies into the project's build file. Dependencies can be managed through Maven or Gradle.
- Define states and events: The states and events required to create a state machine. States represent different states in the system, and events represent transition triggering conditions between states.
- Configuring the state machine: You can use XML configuration files, annotations or programming to configure the state machine. Specify the initial state, state transition rules, and state processing logic.
- Implement state processing: Write state processing logic, that is, code for entering the state, leaving the state, and handling events in the state. State handling methods can be defined by implementing the corresponding interface or using annotations.
- Build a state machine: Use Spring Statemachine's API to build a state machine instance, and apply configuration and state processing logic to the state machine.
- Trigger events: By calling the trigger method of the state machine, an event is sent to trigger the transition between states. The state machine executes corresponding state transition and state processing logic according to configured rules.
- Monitor state changes: You can register a state listener to listen for state change events of the state machine. State listeners can execute custom logic before and after a state transition or when entering a leaving state.
The following is a simple state machine example, showing the flow of an order status:
public enum OrderStatus {
CREATED, PROCESSING, SHIPPED, DELIVERED, CANCELED
}
public enum OrderEvent {
PAYMENT_RECEIVED, PROCESSING_COMPLETE, ITEM_SHIPPED, DELIVERY_CONFIRMED, CANCEL_REQUESTED
}
@Configuration
@EnableStateMachine
public class OrderStateMachineConfig extends EnumStateMachineConfigurerAdapter<OrderStatus, OrderEvent> {
@Override
public void configure(StateMachineTransitionConfigurer<OrderStatus, OrderEvent> transitions) throws Exception {
transitions
.withExternal()
.source(OrderStatus.CREATED)
.target(OrderStatus.PROCESSING)
.event(OrderEvent.PAYMENT_RECEIVED)
.and()
.withExternal()
.source(OrderStatus.PROCESSING)
.target(OrderStatus.SHIPPED)
.event(OrderEvent.PROCESSING_COMPLETE)
.and()
.withExternal()
.source(OrderStatus.SHIPPED)
.target(OrderStatus.DELIVERED)
.event(OrderEvent.ITEM_SHIPPED)
.and()
.withExternal()
.source(OrderStatus.DELIVERED)
.target(OrderStatus.CANCELED)
.event(OrderEvent.CANCEL_REQUESTED);
}
@Override
public void configure(StateMachineConfigurationConfigurer<OrderStatus, OrderEvent> config) throws Exception {
config
.withConfiguration()
.autoStartup(true);
}
@Override
public void configure(StateMachineStateConfigurer<OrderStatus, OrderEvent> states) throws Exception {
states
.withStates()
.initial(OrderStatus.CREATED)
.states(EnumSet.allOf(OrderStatus.class));
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
In the above case, the order status (OrderStatus) and event (OrderEvent) are defined, and then the transition rules between states are configured through StateTransitionConfigurer. The initial state, state transitions, and events that trigger the transitions are specified in the configuration. StateMachineConfigurationConfigurer and StateMachineStateConfigurer are used to configure other properties and initial states of the state machine.
This is just a simple example, the actual state machine may involve more complex state flow and business logic. Complex state transitions and state processing can be easily managed using Spring Statemachine, providing good scalability and flexibility.
Please note that the configuration in the above example is based on annotations. You can also use XML configuration files or programmatically to configure the state machine. The specific configuration method depends on your project needs and personal preferences.