Spring 優雅的串流狀態數據
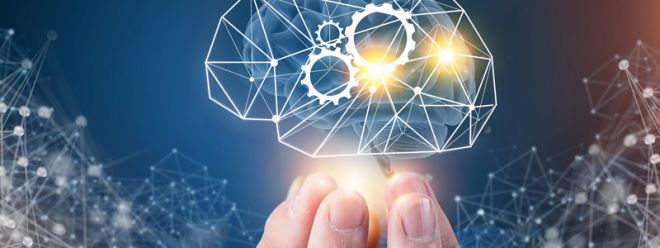
使用Spring Statemachine(狀態機)時,可以按照以下步驟進行使用和實作:
- 引入依賴:在專案的建置文件中引入Spring Statemachine 的依賴。可以透過Maven 或Gradle 來管理依賴。
- 定義狀態和事件:建立狀態機所需的狀態和事件。狀態代表系統中的不同狀態,事件代表狀態之間的轉換觸發條件。
- 設定狀態機:可以使用XML 設定檔、註解或程式設計方式來進行狀態機的設定。指定初始狀態、狀態轉換規則、狀態處理邏輯。
- 實現狀態處理:編寫狀態處理邏輯,即進入狀態、離開狀態和在狀態中處理事件的程式碼。可以透過實作對應的介面或使用註解來定義狀態處理方法。
- 建置狀態機:使用Spring Statemachine 的API 來建置狀態機實例,並將設定和狀態處理邏輯套用到狀態機上。
- 觸發事件:透過呼叫狀態機的觸發方法,發送事件來觸發狀態之間的轉換。狀態機會根據配置的規則執行對應的狀態轉換和狀態處理邏輯。
- 監聽狀態變更:可以註冊狀態監聽器,監聽狀態機的狀態變更事件。狀態監聽器可以在狀態轉換前後或進入離開狀態時執行自訂邏輯。
以下是一個簡單的狀態機案例,展示了一個訂單狀態的流轉:
public enum OrderStatus {
CREATED, PROCESSING, SHIPPED, DELIVERED, CANCELED
}
public enum OrderEvent {
PAYMENT_RECEIVED, PROCESSING_COMPLETE, ITEM_SHIPPED, DELIVERY_CONFIRMED, CANCEL_REQUESTED
}
@Configuration
@EnableStateMachine
public class OrderStateMachineConfig extends EnumStateMachineConfigurerAdapter<OrderStatus, OrderEvent> {
@Override
public void configure(StateMachineTransitionConfigurer<OrderStatus, OrderEvent> transitions) throws Exception {
transitions
.withExternal()
.source(OrderStatus.CREATED)
.target(OrderStatus.PROCESSING)
.event(OrderEvent.PAYMENT_RECEIVED)
.and()
.withExternal()
.source(OrderStatus.PROCESSING)
.target(OrderStatus.SHIPPED)
.event(OrderEvent.PROCESSING_COMPLETE)
.and()
.withExternal()
.source(OrderStatus.SHIPPED)
.target(OrderStatus.DELIVERED)
.event(OrderEvent.ITEM_SHIPPED)
.and()
.withExternal()
.source(OrderStatus.DELIVERED)
.target(OrderStatus.CANCELED)
.event(OrderEvent.CANCEL_REQUESTED);
}
@Override
public void configure(StateMachineConfigurationConfigurer<OrderStatus, OrderEvent> config) throws Exception {
config
.withConfiguration()
.autoStartup(true);
}
@Override
public void configure(StateMachineStateConfigurer<OrderStatus, OrderEvent> states) throws Exception {
states
.withStates()
.initial(OrderStatus.CREATED)
.states(EnumSet.allOf(OrderStatus.class));
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
在上述案例中,定義了訂單的狀態(OrderStatus)和事件(OrderEvent),然後透過 StateTransitionConfigurer 配置了狀態之間的轉換規則。配置中指定了初始狀態、狀態轉換和觸發轉換的事件。 StateMachineConfigurationConfigurer 和StateMachineStateConfigurer 用於設定狀態機的其他屬性和初始狀態。
這只是一個簡單的範例,實際的狀態機可能涉及更複雜的狀態流轉和業務邏輯。使用Spring Statemachine 可以輕鬆管理複雜的狀態轉換和狀態處理,提供了良好的可擴展性和靈活性。
請注意,上述範例中的設定是基於註解方式進行的,你也可以使用XML 設定檔或程式設計方式來設定狀態機。具體的配置方式是根據你的專案需求和個人偏好而定。