Get a complete guide to Python timestamps to better handle time-related tasks
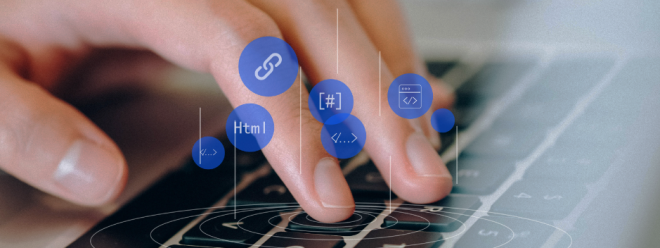
A timestamp is a numerical value that represents a date and time, usually in seconds. Obtaining timestamps is a common task in Python, used for recording events, timing operations, and tracking time in a variety of applications. This article will introduce multiple methods of obtaining timestamps, including standard library and third-party library methods, and provide sample code to help you better understand.
1. Introduction to timestamps
What is a timestamp?
A timestamp is a number that usually represents the number of seconds that have elapsed since a specific date (usually January 1, 1970 at midnight UTC). It is used to record events, track time, and measure time intervals in computer systems.
Timestamp application scenarios
Timestamps are used in a wide variety of applications, including:
- Timing operations: measuring code execution time, performance analysis, etc.
- Logging: Record the time when an event occurs.
- Data storage and processing: Timestamps are used to identify and sort data.
- Cache Control: In web development, timestamps are used to verify whether a resource has changed.
- Time calculation: perform operations and comparisons on dates and times.
- Scheduled tasks: schedule the execution of tasks.
- Data backup: Mark the time point for backup.
2. Use the standard library to obtain timestamps
The Python standard library provides multiple ways to obtain timestamps.
Here are some common methods:
Using the time module
Python's time module provides the time() function to obtain the timestamp of the current time.
import time
timestamp = time.time()
print("当前时间戳:", timestamp)
- 1.
- 2.
- 3.
- 4.
Using the datetime module
The datetime class in the datetime module can be used to get the current date and time and then convert it to a timestamp.
from datetime import datetime
now = datetime.now()
timestamp = datetime.timestamp(now)
print("当前时间戳:", timestamp)
- 1.
- 2.
- 3.
- 4.
- 5.
Use calendar module
The timegm() function in the calendar module can convert UTC time tuples into timestamps.
import calendar
utc_time_tuple = (2023, 10, 24, 12, 0, 0)
timestamp = calendar.timegm(utc_time_tuple)
print("时间戳:", timestamp)
- 1.
- 2.
- 3.
- 4.
- 5.
3. Obtaining timestamps from third-party libraries
In addition to the standard library, there are some popular third-party libraries available for obtaining timestamps.
Use arrow library
Arrow is a powerful third-party library for working with dates and times. It can easily get the current timestamp.
Install the Arrow library:
pip install arrow
- 1.
Then use the following code to get the timestamp:
import arrow
timestamp = arrow.now().timestamp
print("当前时间戳:", timestamp)
- 1.
- 2.
- 3.
- 4.
Use pendulum library
Pendulum is another powerful library for date and time processing. You can use this to get the timestamp.
Install Pendulum library:
pip install pendulum
- 1.
Then use the following code to get the timestamp:
import pendulum
timestamp = pendulum.now().timestamp()
print("当前时间戳:", timestamp)
- 1.
- 2.
- 3.
- 4.
4. Application example of obtaining timestamp
Timing operations
Timestamps are often used to measure code execution time for performance analysis.
Here is an example of using the time module to calculate the execution time of a certain piece of code:
import time
start_time = time.time()
# 执行需要计时的代码
end_time = time.time()
execution_time = end_time - start_time
print("执行时间:", execution_time, "秒")
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
logging
In logging, timestamps are used to identify the point in time when an event occurred for tracking and debugging purposes.
Here is an example of logging using the datetime module:
from datetime import datetime
log_time = datetime.now()
log_message = "Something happened."
log_entry = f"{log_time}: {log_message}"
# 将log_entry写入日志文件
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
Data storage and processing
Timestamps can be used to identify and sort data, especially in databases.
The following example demonstrates how to use the time module to timestamp data:
import time
data = {"value": 42, "timestamp": int(time.time())}
# 存储data到数据库
- 1.
- 2.
- 3.
- 4.
Summarize
This article introduces several methods of obtaining timestamps in Python. Timestamps are important values used to represent dates and times, usually in seconds. Obtaining timestamps is critical in many applications, including performance analysis, logging, data processing, and time calculations.
First, we have an in-depth understanding of the definition and application scenarios of timestamps. Subsequently, the method of using the Python standard library is introduced, including the time , datetime and calendar modules. These methods provide flexibility and precision to meet a variety of needs.
In addition, two popular third-party libraries, Arrow and Pendulum, are introduced, which provide more convenient ways to obtain timestamps and provide more date and time processing functions.
Finally, we provide some application examples, including timing operations, logging, and data storage, to demonstrate the many uses of timestamps in real-world programming.
By mastering these timestamp acquisition methods, you will be able to better handle time-related tasks and improve the readability and maintainability of your code. Timestamps are a powerful tool in Python that help you better manage time and dates, thereby improving programming efficiency. Whether you're performing performance analysis or building time-sensitive applications, understanding these methods will help you in your work.