介面自動化框架裡常用的小工具
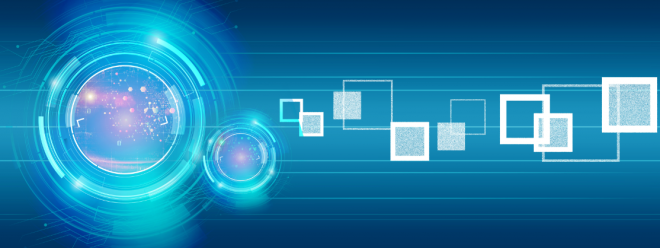
在日常程式設計工作中,我們常常需要處理各種與時間、資料格式及設定檔相關的問題。本文整理了一系列實用的Python程式碼片段,涵蓋了日期時間轉換、資料格式化與轉換、取得檔案註解以及讀取設定檔等內容,助力開發者提升工作效率,輕鬆應對常見任務。
1. 秒級與毫秒級時間戳獲取
# 获取当前秒级时间戳
def millisecond(add=0):
return int(time.time()) + add
# 获取当前毫秒级时间戳
def millisecond_new():
t = time.time()
return int(round(t * 1000))
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
這兩個函數分別提供了取得當前時間的秒級和毫秒級時間戳記的功能。 millisecond()函數允許傳入一個可選參數add,用於增加指定的時間偏移。
2. 當前日期字串獲取
#获取当前时间日期: 20211009
def getNowTime(tianshu=0):
shijian = int(time.strftime('%Y%m%d')) - tianshu
print(shijian)
return shijian
- 1.
- 2.
- 3.
- 4.
- 5.
getNowTime()函數傳回目前日期(格式為YYYYMMDD),並支援傳入參數tianshu以減去指定天數。此函數適用於需要處理日期型資料且僅關注年月日的情況。
3.修復介面返回無引號JSON數據
def json_json():
with open("源文件地址", "r") as f, open("目标文件地址", "a+") as a:
a.write("{")
for line in f.readlines():
if "[" in line.strip() or "{" in line.strip():
formatted_line = "'" + line.strip().replace(":", "':").replace(" ", "") + ","
print(formatted_line) # 输出修复后的行
a.write(formatted_line + "\n")
else:
formatted_line = "'" + line.strip().replace(":", "':'").replace(" ", "") + "',"
print(formatted_line) # 输出修复后的行
a.write(formatted_line + "\n")
a.write("}")
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
此函數用於處理從介面複製的未正確格式化的JSON數據,修復缺少的引號,並將其寫入新的檔案。來源檔案與目標檔案的路徑需替換為實際路徑。
4.將URL查詢字串轉為JSON
from urllib.parse import urlsplit, parse_qs
def query_json(url):
query = urlsplit(url).query
params = dict(parse_qs(query))
cleaned_params = {k: v[0] for k, v in params.items()}
return cleaned_params
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
query_json()函數接收一個包含查詢字串的URL,解析其查詢部分,將其轉換為字典形式,並清理多值參數,只保留第一個值。
5.文件註釋提取
import os
def get_first_line_comments(directory, output_file):
python_files = sorted([f for f in os.listdir(directory) if f.endswith('.py') and f != '__init__.py'])
comments_and_files = []
for file in python_files:
filepath = os.path.join(directory, file)
with open(filepath, 'r', encoding='utf-8') as f:
first_line = f.readline().strip()
if first_line.startswith('#'):
comment = first_line[1:].strip()
comments_and_files.append((file, comment))
with open(output_file, 'w', encoding='utf-8') as out:
for filename, comment in comments_and_files:
out.write(f"{filename}: {comment}\n")
# 示例用法
get_first_line_comments('指定文件夹', '指定生成文件路径.txt')
get_first_line_comments()函数遍历指定目录下的.py文件,提取每份文件的第
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
6.讀取配置INI文件
import sys
import os
import configparser
class ReadConfig:
def __init__(self, config_path):
self.path = config_path
def read_sqlConfig(self, fileName="sql.ini"):
read_mysqlExecuteCon = configparser.ConfigParser()
read_mysqlExecuteCon.read(os.path.join(self.path, fileName), encoding="utf-8")
return read_mysqlExecuteCon._sections
def read_hostsConfig(self, fileName="hosts.ini"):
read_hostsCon = configparser.ConfigParser()
read_hostsCon.read(os.path.join(self.path, fileName), encoding="utf-8")
return read_hostsCon._sections
# 示例用法
config_reader = ReadConfig('配置文件所在路径')
sql_config = config_reader.read_sqlConfig()
hosts_config = config_reader.read_hostsConfig()["hosts"]
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
7.設定全域檔案路徑
import os
def setFilePath(filePath):
current_module_path = os.path.dirname(os.path.abspath(__file__))
project_root_path = os.path.dirname(os.path.dirname(current_module_path))
path = os.path.join(project_root_path, filePath.lstrip('/'))
return os.path.abspath(path)
# 示例用法
confPath = setFilePath("地址文件路径")
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
setFilePath()函數根據提供的相對路徑,結合目前模組的絕對路徑,計算出專案根目錄下的目標檔案或目錄的絕對路徑,以便在專案中統一管理資源位置。