JS小知識,如何將CSV 轉換為JSON 字串
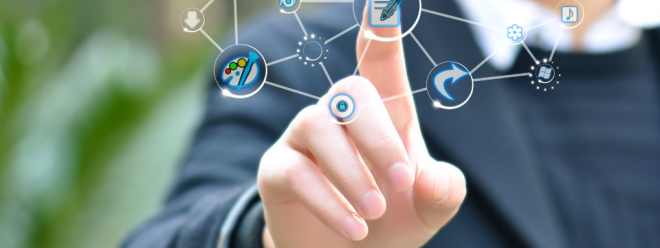
使用csvtojson 第三方函式庫
您可以使用 csvtojson 庫在JavaScript 中快速將CSV 轉換為JSON 字串:
索引.js
import csvToJson from 'csvtojson';
const csvFilePath = 'data.csv';
const json = await csvToJson().fromFile(csvFilePath);
console.log(json);
- 1.
- 2.
- 3.
- 4.
data.csv 文件
例如這樣的data.csv 文件,其內容如下:
color,maxSpeed,age
"red",120,2
"blue",100,3
"green",130,2
- 1.
- 2.
- 3.
- 4.
最終產生的JSON 陣列字串內容如下:
[
{ color: 'red', maxSpeed: '120', age: '2' },
{ color: 'blue', maxSpeed: '100', age: '3' },
{ color: 'green', maxSpeed: '130', age: '2' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
安裝csvtojson
在使用csvtojson 之前,您需要將其安裝到我們的專案中。您可以使用NPM 或Yarn CLI 執行此操作。
npm i csvtojson
# Yarn
yarn add csvtojson
- 1.
- 2.
- 3.
- 4.
安裝後,將其引入到你的專案中,如下所示:
import csvToJson from 'csvtojson';
// CommonJS
const csvToJson = require('csvtojson');
- 1.
- 2.
- 3.
- 4.
透過fromFile() 方法,導入CSV文件
我們呼叫csvtojson 模組的預設導出函數來建立將轉換CSV 的物件。這個物件有一堆方法,每個方法都以某種方式與CSV 到JSON 的轉換相關,fromFile() 就是其中之一。
它接受要轉換的CSV 檔案的名稱,並傳回一個Promise,因為轉換是一個非同步過程。Promise 將使用產生的JSON 字串進行解析。
直接將CSV 字串轉換為JSON,fromString()
要直接從CSV 資料字串而不是檔案轉換,您可以使用轉換物件的非同步fromString() 方法來代替:
索引.js
import csvToJson from 'csvtojson';
const csv = `"First Name","Last Name","Age"
"Russell","Castillo",23
"Christy","Harper",35
"Eleanor","Mark",26`;
const json = await csvToJson().fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
[
{ 'First Name': 'Russell', 'Last Name': 'Castillo', Age: '23' },
{ 'First Name': 'Christy', 'Last Name': 'Harper', Age: '35' },
{ 'First Name': 'Eleanor', 'Last Name': 'Mark', Age: '26' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
自訂CSV 到JSON 的轉換
csvtojson 的預設導出函數接受一個對象,用於指定選項,可以自訂轉換過程。
其中一個選項是header,這是一個用於指定CSV 資料中的標題的數組,可以將其替換成更易讀的別名。
索引.js
import csvToJson from 'csvtojson';
const csv = `"First Name","Last Name","Age"
"Russell","Castillo",23
"Christy","Harper",35
"Eleanor","Mark",26`;
const json = await csvToJson({
headers: ['firstName', 'lastName', 'age'],
}).fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
[
{ firstName: 'Russell', lastName: 'Castillo', age: '23' },
{ firstName: 'Christy', lastName: 'Harper', age: '35' },
{ firstName: 'Eleanor', lastName: 'Mark', age: '26' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
另一個是delimeter,用來表示分隔列的字元:
import csvToJson from 'csvtojson';
const csv = `"First Name"|"Last Name"|"Age"
"Russell"|"Castillo"|23
"Christy"|"Harper"|35
"Eleanor"|"Mark"|26`;
const json = await csvToJson({
headers: ['firstName', 'lastName', 'age'],
delimiter: '|',
}).fromString(csv);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
輸出:
[
{ firstName: 'Russell', lastName: 'Castillo', age: '23' },
{ firstName: 'Christy', lastName: 'Harper', age: '35' },
{ firstName: 'Eleanor', lastName: 'Mark', age: '26' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
我們也可以使用ignoreColumns 屬性,一個使用正規表示式示忽略某些列的選項。
import csvToJson from 'csvtojson';
const csv = `"First Name"|"Last Name"|"Age"
"Russell"|"Castillo"|23
"Christy"|"Harper"|35
"Eleanor"|"Mark"|26`;
const json = await csvToJson({
headers: ['firstName', 'lastName', 'age'],
delimiter: '|',
ignoreColumns: /lastName/,
}).fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
將CSV 轉換為行數組
透過將輸出選項設為“csv”,我們可以產生一個數組列表,其中每個數組代表一行,包含該行所有列的值。
如下圖所示:
索引.js
import csvToJson from 'csvtojson';
const csv = `color,maxSpeed,age
"red",120,2
"blue",100,3
"green",130,2`;
const json = await csvToJson({
output: 'csv',
}).fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
[
[ 'red', '120', '2' ],
[ 'blue', '100', '3' ],
[ 'green', '130', '2' ]
]
- 1.
- 2.
- 3.
- 4.
- 5.
使用原生的JS處理CSV 轉JSON
我們也可以在不使用任何第三方函式庫的情況下將CSV 轉換為JSON。
索引.js
function csvToJson(csv) {
// \n or \r\n depending on the EOL sequence
const lines = csv.split('\n');
const delimeter = ',';
const result = [];
const headers = lines[0].split(delimeter);
for (const line of lines) {
const obj = {};
const row = line.split(delimeter);
for (let i = 0; i < headers.length; i++) {
const header = headers[i];
obj[header] = row[i];
}
result.push(obj);
}
// Prettify output
return JSON.stringify(result, null, 2);
}
const csv = `color,maxSpeed,age
"red",120,2
"blue",100,3
"green",130,2`;
const json = csvToJson(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
輸出:
[
{
"color": "color",
"maxSpeed": "maxSpeed",
"age": "age"
},
{
"color": "\"red\"",
"maxSpeed": "120",
"age": "2"
},
{
"color": "\"blue\"",
"maxSpeed": "100",
"age": "3"
},
{
"color": "\"green\"",
"maxSpeed": "130",
"age": "2"
}
]
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
您可以完善上面的程式碼處理更為複雜的CSV 資料。
結束
今天的分享就到這裡,如何將CSV 轉換為JSON 字串,你學會了嗎?