MQTT - a lightweight communication protocol based on publish/subscribe model
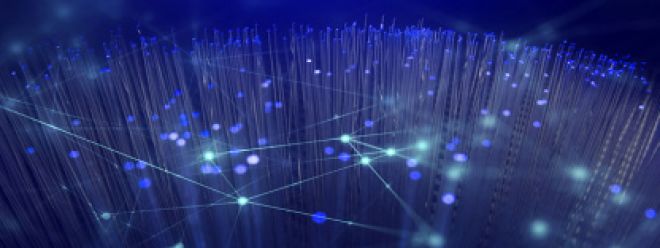
MQTT - a lightweight communication protocol based on publish/subscribe model
Introduction to MQTT
MQTT (Message Queuing Telemetry Transport) is a lightweight communication protocol based on the publish/subscribe model, which is commonly used for communication between IoT devices. It has the following characteristics:
- Simplicity: The MQTT protocol has a simple design and is easy to implement and deploy.
- Lightweight: The protocol header information is small and suitable for use in network environments with limited bandwidth.
- Publish/subscribe mode: supports publishers to publish messages to specific topics, and subscribers can selectively subscribe to topics of interest to achieve message distribution and reception.
- Reliability: Supports three quality of service levels (QoS), including at-most-once, at-least-once and exactly-once message delivery guarantees.
The message format of the MQTT protocol is as follows:
[
Fixed Head Variable Head Payload Fixed Length Variable Length Variable Length
]
Among them, the fixed header contains control message type, flag bit and remaining length field; the variable header contains protocol name, protocol version, connection flag bit and other information; the payload contains the actual message content.
The MQTT protocol is suitable for reliable communication in resource-constrained devices and network environments.
Application scenarios
- IoT device communication: MQTT can be used to connect and communicate with various IoT devices, including sensors, actuators, embedded devices, etc., to achieve data exchange and control between devices. For example, sensor nodes can send data to a cloud server through the MQTT protocol, or devices can communicate in real time through MQTT.
- Remote monitoring and control: MQTT can be used for remote monitoring and control systems, such as remote monitoring of factory equipment, smart home equipment, agricultural automation systems, etc.
- Real-time data transmission: MQTT supports publish/subscribe mode and can be used to transmit data in real time, such as weather data, traffic information, stock quotes, etc.
- Mobile application notifications: MQTT can be used to send real-time notifications to mobile applications, such as social media updates, news alerts, instant messages, etc.
- Resource-constrained environments: Due to the lightweight nature of the MQTT protocol, it is suitable for use in resource-constrained environments, such as embedded systems, sensor networks, and low-bandwidth network environments.
MQTT is suitable for various IoT and real-time data transmission scenarios that require lightweight, reliable, and real-time communication.
Protocol description
MQTT control message format
- "Fixed Header": Contains message type and flag bits.
- "Variable Header": Varies according to different message types and contains some additional information.
- "Payload": Contains the actual data content.
MQTT packet type:
- "CONNECT": The type of data packet sent when the client connects to the server.
- "CONNACK": The server's response packet type to the client's connection request.
- "PUBLISH": The packet type used to publish messages.
- "PUBACK": Confirmation packet type for PUBLISH message.
- "PUBREC, PUBREL, PUBCOMP": QoS level 2 packet types used to publish messages.
- "SUBSCRIBE": The packet type of the subscription topic.
- "SUBACK": Confirmation packet type for subscription request.
- "UNSUBSCRIBE": The packet type to unsubscribe from the topic.
- "UNSUBACK": Confirmation packet type for unsubscription request.
- "PINGREQ, PINGRESP": The data packet type used to maintain the connection.
- "DISCONNECT": The type of data packet sent when the client disconnects.
Each packet type has a specific format and purpose for communication and messaging in the MQTT protocol.
MQTT message flags:
- "Retain": Indicates whether the server should retain the message.
- "QoS Level": Specifies the quality of message delivery.
- "Topic Subscription Identifier": Indicates whether to use a topic subscription identifier.
The format of a PUBLISH message is as follows:
| 固定报头 | 可变报头 | 有效载荷 |
- 1.
Among them, the fixed header contains the message type and flag bit, the variable header contains the subject name and message identifier, and the payload contains the actual message content.
MQTT use
Import dependencies:
repositories {
maven {
url "https://repo.eclipse.org/content/repositories/paho-snapshots/"
}
}
dependencies {
compile 'org.eclipse.paho:org.eclipse.paho.client.mqttv3:1.1.0'
compile 'org.eclipse.paho:org.eclipse.paho.android.service:1.1.1'
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
Create a client and publish messages (the server needs to build an MQTT service):
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
public class MqttJavaExample {
public static void main(String[] args) {
String broker = "tcp://iot.eclipse.org:1883";
String clientId = "JavaExample";
try {
MqttClient client = new MqttClient(broker, clientId);
MqttConnectOptions connOpts = new MqttConnectOptions();
connOpts.setCleanSession(true);
System.out.println("Connecting to broker: " + broker);
client.connect(connOpts);
System.out.println("Connected");
String topic = "iot/topic";
String content = "Hello, MQTT!";
int qos = 2;
MqttMessage message = new MqttMessage(content.getBytes());
message.setQos(qos);
System.out.println("Publishing message: " + content);
client.publish(topic, message);
System.out.println("Message published");
client.disconnect();
System.out.println("Disconnected");
System.exit(0);
} catch (MqttException me) {
System.out.println("reason " + me.getReasonCode());
System.out.println("msg " + me.getMessage());
System.out.println("loc " + me.getLocalizedMessage());
System.out.println("cause " + me.getCause());
System.out.println("excep " + me);
me.printStackTrace();
}
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
This is a simple example using the Eclipse Paho MQTT Java client library.
import org.eclipse.paho.client.mqttv3.*;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
public class MqttClientExample {
public static void main(String[] args) {
String broker = "tcp://iot.eclipse.org:1883";
String clientId = "JavaSample";
MemoryPersistence persistence = new MemoryPersistence();
try {
MqttClient client = new MqttClient(broker, clientId, persistence);
MqttConnectOptions connOpts = new MqttConnectOptions();
connOpts.setCleanSession(true);
System.out.println("Connecting to broker: " + broker);
client.connect(connOpts);
System.out.println("Connected");
String topic = "iot/topic";
int qos = 2;
client.subscribe(topic, qos);
System.out.println("Subscribed to topic: " + topic);
String content = "Hello, MQTT!";
MqttMessage message = new MqttMessage(content.getBytes());
message.setQos(qos);
client.publish(topic, message);
System.out.println("Message published");
client.disconnect();
System.out.println("Disconnected");
System.exit(0);
} catch (MqttException me) {
System.out.println("reason " + me.getReasonCode());
System.out.println("msg " + me.getMessage());
System.out.println("loc " + me.getLocalizedMessage());
System.out.println("cause " + me.getCause());
System.out.println("excep " + me);
me.printStackTrace();
}
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
This is a simple example using the Eclipse Paho MQTT Java client. In this example, we create an MQTT client and connect to the specified MQTT broker, then subscribe to a topic and publish a message.
MQTT advantages and disadvantages
advantage
- "Lightweight Protocol": MQTT is a lightweight publish/subscribe message transmission protocol suitable for restricted network environments and devices.
- "Low bandwidth consumption": Due to its streamlined protocol header and binary message format, MQTT consumes low bandwidth during transmission.
- "Reliability": MQTT supports three levels of quality of service (QoS). You can choose the appropriate level according to your needs to ensure reliable transmission of messages.
- "Flexibility": MQTT supports message transmission in a variety of scenarios, including communication between the device and the cloud, mobile application notification push, etc.
- "Easy to integrate": The MQTT protocol has been widely supported, and there are many open source client and server implementations that are easy to integrate into various applications.
shortcoming
- "Security": MQTT itself does not provide encryption functions, and requires TLS/SSL and other methods to ensure communication security.
- "Complexity": In some specific scenarios, such as the need to implement advanced message queue functions or large-scale deployment, complex configuration and management may be required.
- "Not suitable for big data transmission": Due to its lightweight characteristics, MQTT is not suitable for large-scale data transmission and is suitable for transmitting small control information and sensor data.
- "Requires a stable network connection": Due to its TCP-based nature, MQTT requires a stable network connection to ensure reliable transmission of messages, and is not suitable for use in environments with unstable networks.