Routing model of RabbitMQ communication model
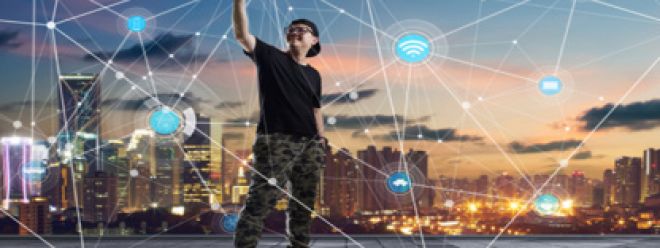
Routing model of RabbitMQ communication model
Hello everyone, I am amazing.
Today, I am going to lead you to learn RabbitMQ and understand the routing model, one of the five major communication models of RabbitMQ; there will be a series of tutorials about RabbitMQ in the future, remember to pay attention if it is helpful to you~
Past Portal
RabbitMQ (一)hello world
RabbitMQ (2) work model
RabbitMQ (3) Publish-Subscribe Model
routing model
RabbitMQ provides five different communication models. In the previous article, I briefly introduced RabbitMQ's publish and subscribe model. This article is to learn about the routing model (direct) in RabbitMQ.
Routing model (direct): The routing model is equivalent to an upgraded version of the distributed subscription model, with an additional routing key to constrain the binding of queues and switches.
In the routing model, the producer sends the message to the exchange, and the exchange forwards the message to the corresponding queue according to the routing key of the message. Each queue can be bound to multiple routing keys, and each routing key can be bound to multiple queues. Consumers receive messages from the queue and process them. When a routing key is bound to multiple queues, the exchange will send the message to all bound queues. When a queue is bound to multiple routing keys, the queue will be able to receive messages corresponding to all routing keys.
Applicable scene
The routing model is suitable for scenarios that require point-to-point communication, such as:
- System monitoring alarm notification;
- task distribution;
- User private message system;
- Order confirmation notification, etc.
demo
- producer
// 生产者
public class Producer {
private static final String EXCHANGE_NAME = "exchange_direct_1";
// 定义路由的key,key值是可以随意定义的
private static final String EXCHANGE_ROUTING_KEY1 = "direct_km1";
private static final String EXCHANGE_ROUTING_KEY2 = "direct_km2";
public static void main(String[] args) throws IOException, TimeoutException {
Connection connection = ConnectionUtils.getConnection();
Channel channel = connection.createChannel();
channel.exchangeDeclare(EXCHANGE_NAME, "direct");
for (int i = 0; i < 100; i++) {
if (i % 2 == 0) {
channel.basicPublish(EXCHANGE_NAME, EXCHANGE_ROUTING_KEY1, null, ("路由模型发送的第 " + i + " 条信息").getBytes());
} else {
channel.basicPublish(EXCHANGE_NAME, EXCHANGE_ROUTING_KEY2, null, ("路由模型发送的第 " + i + " 条信息").getBytes());
}
}
channel.close();
connection.close();
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- consumer
// 消费者1
public class Consumer {
private static final String QUEUE_NAME = "queue_direct_1";
private static final String EXCHANGE_NAME = "exchange_direct_1";
private static final String EXCHANGE_ROUTING_KEY1 = "direct_km1";
public static void main(String[] args) throws IOException, TimeoutException {
Connection connection = ConnectionUtils.getConnection();
Channel channel = connection.createChannel();
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
channel.exchangeDeclare(EXCHANGE_NAME, "direct");
channel.queueBind(QUEUE_NAME, EXCHANGE_NAME, EXCHANGE_ROUTING_KEY1);
DefaultConsumer defaultConsumer = new DefaultConsumer(channel) {
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("消费者1接收到的消息是:" + new String(body));
}
};
channel.basicConsume(QUEUE_NAME, true, defaultConsumer);
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
// 消费者2
public class Consumer2 {
private static final String QUEUE_NAME = "queue_direct_2";
private static final String EXCHANGE_NAME = "exchange_direct_1";
private static final String EXCHANGE_ROUTING_KEY2 = "direct_km2";
public static void main(String[] args) throws IOException, TimeoutException {
Connection connection = ConnectionUtils.getConnection();
Channel channel = connection.createChannel();
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
channel.exchangeDeclare(EXCHANGE_NAME, "direct");
channel.queueBind(QUEUE_NAME, EXCHANGE_NAME, EXCHANGE_ROUTING_KEY2);
DefaultConsumer defaultConsumer = new DefaultConsumer(channel) {
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("消费者2接收到的消息是:" + new String(body));
}
};
channel.basicConsume(QUEUE_NAME, true, defaultConsumer);
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- The test
starts 2 consumers first, and then starts the producer
to get the result that consumer 1 gets a message with an even number and
consumer 2 gets a message with an odd number
summary
This article introduces the use of the routing model in the RabbitMQ communication model, which realizes point-to-point communication through switches and routing keys, which is suitable for scenarios that require point-to-point communication. In actual use, you need to pay attention to the following points:
- The routing key must be the same as the routing key when the consumer binds the queue, otherwise the message cannot be received;
- More flexible message routing can be achieved through multiple exchanges and routing keys.
In the follow-up, it will continue to update the RabbitMQ series of articles, and interested friends will continue to pay attention~~~