How does SpringBoot ensure interface security? That's what old birds do!
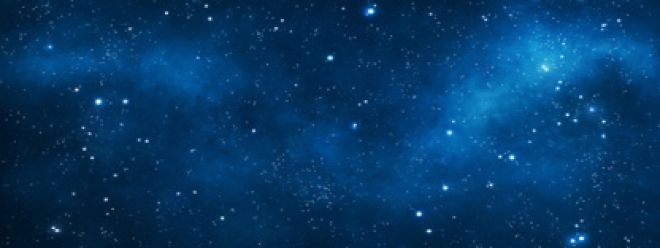
How does SpringBoot ensure interface security? That's what old birds do!
Hello everyone, I am Piao Miao.
For the Internet, as long as the interface of your system is exposed to the external network, interface security issues cannot be avoided. If your interface is running naked on the external network, as long as you know the address and parameters of the interface, you can call it, it will be a disaster.
For example: when your website user registers, you need to fill in your mobile phone number and send a mobile phone verification code. If the interface for sending the verification code has not undergone special security treatment, then this SMS interface has long been stolen, and I don't know how much money is wasted. up.
So how to ensure the security of the interface?
Generally speaking, the API interface exposed on the external network needs to be tamper-proof and anti-replay before it can be called a secure interface.
tamper proof
We know that http is a stateless protocol. The server does not know whether the request sent by the client is legal, nor does it know whether the parameters in the request are correct.
For example, now there is a recharge interface, which can increase the corresponding balance for the user after calling.
http://localhost/api/user/recharge?user_id=1001&amount=10
- 1.
If the illegal user obtains the interface parameters through packet capture, modifying the value of user_id or amount can achieve the purpose of adding balance to any account.
How to solve
The transmitted plaintext can be encrypted by using the HTTPS protocol, but hackers can still intercept the transmitted data packets and further forge requests for replay attacks. If the hacker uses a special method to make the requesting device use a forged certificate to communicate, the HTTPS encrypted content will also be decrypted.
There are 2 general approaches:
- The data of the interface is encrypted and transmitted in https mode. Even if it is cracked by hackers, hackers will spend a lot of time and energy to crack it.
- The interface background verifies the request parameters of the interface to prevent tampering by hackers;
- Step 1: The client encrypts the transmitted parameters with the agreed secret key, obtains the signature value sign1, and puts the signature value into the request parameters, and sends the request to the server
- Step 2: The server receives the client's request, and then uses the agreed secret key to sign the request parameters again to obtain the signature value sign2.
- Step 3: The server compares the values of sign1 and sign2, and if they are inconsistent, it is considered tampered and an illegal request.
anti-replay
Anti-replay is also called anti-multiplexing. To put it simply, I did not change anything after obtaining the requested information, and directly took the parameters of the interface to repeatedly request the recharge interface. At this point my request is legal, because all the parameters are exactly the same as the legal request. A replay attack has two consequences:
- For inserting database interface: replay attack, there will be a lot of duplicate data, and even garbage data will burst the database.
- Interfaces for queries: Hackers generally focus on attacking slow query interfaces. For example, a slow query interface 1s, as long as the hacker launches a replay attack, the system will inevitably be dragged down and the database query will be blocked to death.
There are generally two approaches to replay attacks:
Timestamp-based scheme
For each HTTP request, the timestamp parameter needs to be added, and then the timestamp and other parameters are digitally signed. Because a normal HTTP request generally does not exceed 60s from sending to the server, so after the server receives the HTTP request, it first judges whether the timestamp parameter exceeds 60s compared with the current time, and if it exceeds, it is considered an illegal request.
Under normal circumstances, the hacker takes far more than 60s to replay the request from the captured packet, so the timestamp parameter in the request is invalid at this time. If the hacker modifies the timestamp parameter to the current timestamp, the digital signature corresponding to the sign1 parameter will become invalid, because the hacker does not know the signature key and cannot generate a new digital signature.
However, the loophole of this method is also obvious. If a replay attack is carried out within 60s, there is no way, so this method cannot guarantee that the request is valid only once.
Veterans generally adopt the following solution, which can not only solve the problem of interface replay, but also solve the problem of valid interface request once.
Scheme based on nonce + timestamp
nonce means a random string that is only valid once, and requires that the parameter be different for each request. After actually using information such as user information + timestamp + random number to make a hash, use it as the nonce parameter.
At this time, the processing flow of the server is as follows:
- Go to redis to find out if there is a string whose key is nonce:{nonce}
- If not, create this key, and set the expiration time of this key to be the same as the expiration time of the verification timestamp, for example, 60s.
- If so, it means that the key has been used within 60s, and this request can be judged as a replay request.
Both the nonce and timestamp parameters of this scheme are passed to the backend as part of the signature. Based on the timestamp scheme, hackers can only carry out replay attacks within 60 seconds. After adding the nonce random number, it can ensure that the interface can only be called once, which can be very fast. Good solution to the replay attack problem.
Code
Next, let's see how to implement anti-tampering and anti-replay of the interface through the actual code.
1. Build the request header object
@Data
@Builder
public class RequestHeader {
private String sign ;
private Long timestamp ;
private String nonce;
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
2. The tool class obtains request parameters from HttpServletRequest
@Slf4j
@UtilityClass
public class HttpDataUtil {
/**
* post请求处理:获取 Body 参数,转换为SortedMap
*
* @param request
*/
public SortedMap<String, String> getBodyParams(final HttpServletRequest request) throws IOException {
byte[] requestBody = StreamUtils.copyToByteArray(request.getInputStream());
String body = new String(requestBody);
return JsonUtil.json2Object(body, SortedMap.class);
}
/**
* get请求处理:将URL请求参数转换成SortedMap
*/
public static SortedMap<String, String> getUrlParams(HttpServletRequest request) {
String param = "";
SortedMap<String, String> result = new TreeMap<>();
if (StringUtils.isEmpty(request.getQueryString())) {
return result;
}
try {
param = URLDecoder.decode(request.getQueryString(), "utf-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
String[] params = param.split("&");
for (String s : params) {
String[] array=s.split("=");
result.put(array[0], array[1]);
}
return result;
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
The parameters here are placed in the SortedMap to sort them lexicographically, and the front-end also needs to sort the parameters lexicographically when building a signature.
3. Signature verification tools
@Slf4j
@UtilityClass
public class SignUtil {
/**
* 验证签名
* 验证算法:把timestamp + JsonUtil.object2Json(SortedMap)合成字符串,然后MD5
*/
@SneakyThrows
public boolean verifySign(SortedMap<String, String> map, RequestHeader requestHeader) {
String params = requestHeader.getNonce() + requestHeader.getTimestamp() + JsonUtil.object2Json(map);
return verifySign(params, requestHeader);
}
/**
* 验证签名
*/
public boolean verifySign(String params, RequestHeader requestHeader) {
log.debug("客户端签名: {}", requestHeader.getSign());
if (StringUtils.isEmpty(params)) {
return false;
}
log.info("客户端上传内容: {}", params);
String paramsSign = DigestUtils.md5DigestAsHex(params.getBytes()).toUpperCase();
log.info("客户端上传内容加密后的签名结果: {}", paramsSign);
return requestHeader.getSign().equals(paramsSign);
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
4. HttpServletRequest wrapper class
public class SignRequestWrapper extends HttpServletRequestWrapper {
//用于将流保存下来
private byte[] requestBody = null;
public SignRequestWrapper(HttpServletRequest request) throws IOException {
super(request);
requestBody = StreamUtils.copyToByteArray(request.getInputStream());
}
@Override
public ServletInputStream getInputStream() throws IOException {
final ByteArrayInputStream bais = new ByteArrayInputStream(requestBody);
return new ServletInputStream() {
@Override
public boolean isFinished() {
return false;
}
@Override
public boolean isReady() {
return false;
}
@Override
public void setReadListener(ReadListener readListener) {
}
@Override
public int read() throws IOException {
return bais.read();
}
};
}
@Override
public BufferedReader getReader() throws IOException {
return new BufferedReader(new InputStreamReader(getInputStream()));
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
We will implement anti-tampering and anti-replay through SpringBoot Filter, and the filter filter we write needs to read the request data stream, but the request data stream can only be read once, and we need to implement HttpServletRequestWrapper to wrap the data stream by ourselves. The stream is saved.
5. Create a filter to implement security verification
@Configuration
public class SignFilterConfiguration {
@Value("${sign.maxTime}")
private String signMaxTime;
//filter中的初始化参数
private Map<String, String> initParametersMap = new HashMap<>();
@Bean
public FilterRegistrationBean contextFilterRegistrationBean() {
initParametersMap.put("signMaxTime",signMaxTime);
FilterRegistrationBean registration = new FilterRegistrationBean();
registration.setFilter(signFilter());
registration.setInitParameters(initParametersMap);
registration.addUrlPatterns("/sign/*");
registration.setName("SignFilter");
// 设置过滤器被调用的顺序
registration.setOrder(1);
return registration;
}
@Bean
public Filter signFilter() {
return new SignFilter();
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
@Slf4j
public class SignFilter implements Filter {
@Resource
private RedisUtil redisUtil;
//从fitler配置中获取sign过期时间
private Long signMaxTime;
private static final String NONCE_KEY = "x-nonce-";
@Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
HttpServletRequest httpRequest = (HttpServletRequest) servletRequest;
HttpServletResponse httpResponse = (HttpServletResponse) servletResponse;
log.info("过滤URL:{}", httpRequest.getRequestURI());
HttpServletRequestWrapper requestWrapper = new SignRequestWrapper(httpRequest);
//构建请求头
RequestHeader requestHeader = RequestHeader.builder()
.nonce(httpRequest.getHeader("x-Nonce"))
.timestamp(Long.parseLong(httpRequest.getHeader("X-Time")))
.sign(httpRequest.getHeader("X-Sign"))
.build();
//验证请求头是否存在
if(StringUtils.isEmpty(requestHeader.getSign()) || ObjectUtils.isEmpty(requestHeader.getTimestamp()) || StringUtils.isEmpty(requestHeader.getNonce())){
responseFail(httpResponse, ReturnCode.ILLEGAL_HEADER);
return;
}
/*
* 1.重放验证
* 判断timestamp时间戳与当前时间是否操过60s(过期时间根据业务情况设置),如果超过了就提示签名过期。
*/
long now = System.currentTimeMillis() / 1000;
if (now - requestHeader.getTimestamp() > signMaxTime) {
responseFail(httpResponse,ReturnCode.REPLAY_ERROR);
return;
}
//2. 判断nonce
boolean nonceExists = redisUtil.hasKey(NONCE_KEY + requestHeader.getNonce());
if(nonceExists){
//请求重复
responseFail(httpResponse,ReturnCode.REPLAY_ERROR);
return;
}else {
redisUtil.set(NONCE_KEY+requestHeader.getNonce(), requestHeader.getNonce(), signMaxTime);
}
boolean accept;
SortedMap<String, String> paramMap;
switch (httpRequest.getMethod()){
case "GET":
paramMap = HttpDataUtil.getUrlParams(requestWrapper);
accept = SignUtil.verifySign(paramMap, requestHeader);
break;
case "POST":
paramMap = HttpDataUtil.getBodyParams(requestWrapper);
accept = SignUtil.verifySign(paramMap, requestHeader);
break;
default:
accept = true;
break;
}
if (accept) {
filterChain.doFilter(requestWrapper, servletResponse);
} else {
responseFail(httpResponse,ReturnCode.ARGUMENT_ERROR);
return;
}
}
private void responseFail(HttpServletResponse httpResponse, ReturnCode returnCode) {
ResultData<Object> resultData = ResultData.fail(returnCode.getCode(), returnCode.getMessage());
WebUtils.writeJson(httpResponse,resultData);
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {
String signTime = filterConfig.getInitParameter("signMaxTime");
signMaxTime = Long.parseLong(signTime);
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
- 63.
- 64.
- 65.
- 66.
- 67.
- 68.
- 69.
- 70.
- 71.
- 72.
- 73.
- 74.
- 75.
- 76.
- 77.
- 78.
- 79.
- 80.
- 81.
- 82.
- 83.
- 84.
- 85.
- 86.
- 87.
- 88.
6. Redis Tools
@Component
public class RedisUtil {
@Resource
private RedisTemplate<String, Object> redisTemplate;
/**
* 判断key是否存在
* @param key 键
* @return true 存在 false不存在
*/
public boolean hasKey(String key) {
try {
return Boolean.TRUE.equals(redisTemplate.hasKey(key));
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
/**
* 普通缓存放入并设置时间
* @param key 键
* @param value 值
* @param time 时间(秒) time要大于0 如果time小于等于0 将设置无限期
* @return true成功 false 失败
*/
public boolean set(String key, Object value, long time) {
try {
if (time > 0) {
redisTemplate.opsForValue().set(key, value, time, TimeUnit.SECONDS);
} else {
set(key, value);
}
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
/**
* 普通缓存放入
* @param key 键
* @param value 值
* @return true成功 false失败
*/
public boolean set(String key, Object value) {
try {
redisTemplate.opsForValue().set(key, value);
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.