Real-time chat room WebSocket combat
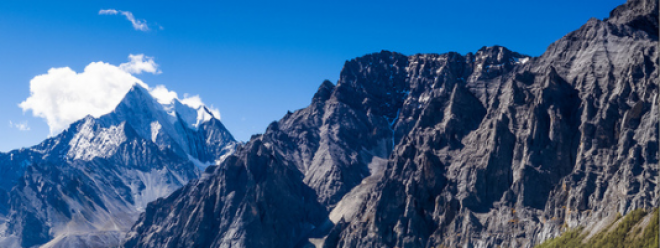
To learn more about open source, please visit :
51CTO Open Source Basic Software Community
foreword
If you want to achieve the same function as WeChat chat, it is obviously not enough to communicate in the network, so the soft bus does not work with this kind of long-distance transmission. If we want to complete the chat function of WeChat, the traditional method is to use webSocket for full-duplex communication with the server.
What is WebSocket?
WebSocket is a network communication protocol for full-duplex communication over a single TCP connection.
When there was no webSocket in the past, everyone used the HTTP protocol for network communication, but the HTTP protocol is a stateless, connectionless, one-way application layer protocol, so the client can only make one-way requests to the server, and the server The inability to proactively send messages to clients makes it difficult to carry out a business like live chat.
Some developers use HTTP for long polling, which means that HTTP must keep connecting requests for a period of time to obtain the latest server information. This is obviously inefficient and very wasteful of resources.
Therefore, WebSocket was born, and it only needs to connect once, and it can maintain the full-duplex communication state all the time.
Demo display
Let's use the officially provided WebSocket interface to implement a demo of a simple real-time chat room. The effect is as follows:
Code
You can see the interface description of the official document, we only need to use a few interfaces to achieve our business needs.
https://developer.harmonyos.com/cn/docs/documentation/doc-guides/websocket-connection-0000001333321005.
1. Apply for network permission
Register network permissions in the config.json file, which allow programs to open network sockets for network connections.
"reqPermissions": [
{
"name": "ohos.permission.INTERNET"
}
]
- 1.
- 2.
- 3.
- 4.
- 5.
2. Import the webSocket module
import webSocket from '@ohos.net.webSocket';
- 1.
3. Create a webSocket object
Then we call the createWebSocket() interface to generate a webSocket object and save it.
let ws = webSocket.createWebSocket();
- 1.
4. Connect to the webSocket channel
Call the connect() interface to connect. Here, a URL is required as a parameter to be passed in. In this demo, a previously developed server interface is directly used for calling, but it is not open to the public, so you only need to put the interface address developed by yourself into "wsURL". Can.
onInit() {
let that = this;
ws.connect("wsURL", (err, value) => {
if (!err) {
console.log("xxx---connect success");
} else {
console.log("xxx---connect fail, err:" + JSON.stringify(err));
}
});
},
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
5. Subscribe to news updates in the channel
Here we call the on( type: 'message' ) interface to monitor the message. It should be noted here that the server passes the string type, so if the message is a JSON object, you need to use JSON.parse() to parse and restore into a JSON object.
onInit() {
let that = this;
ws.on('message', (err, value) => {
console.log("xxx---on message, message:" + value);
//传递的是序列化后的字符串,需要解序列化为JSON对象
let dataObj = JSON.parse(value)
console.log("xxx---parse success---postId: "+dataObj.postId+",message:"+dataObj.message)
that.message.push(dataObj)
console.log("xxx---check message: "+JSON.stringify(that.message))
});
},
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
6. Send a message
Then call the send() interface to send the message. Note here that if the JSON object is to be passed, use JSON.stringify() to serialize it to ensure that we pass it in the form of a stream string.
In the callback of this interface, we can also print it out to see if the message was sent successfully.
sendMessage(){
let that = this;
let data = {
postId:that.id,
message:that.sendMes
}
let dataStr = JSON.stringify(data)
ws.send(dataStr, (err, value) => {
if (!err) {
console.log("xxx---send success");
} else {
console.log("xxx---send fail, err:" + JSON.stringify(err));
}
});
that.message.push(data)
},
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
7. Hide the title bar
Careful friends will find that the black title bar displayed by my demo is gone. In fact, it can be hidden. Just add a few lines of code under module.abilities in the config.json file.
"metaData":{
"customizeData":[
{
"name": "hwc-theme",
"value": "androidhwext:style/Theme.Emui.NoTitleBar",
"extra":""
}
]
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
8. Style Design
The next step is to simply design the interface style, and render the obtained message.
<div class="container">
<div style="width: 100%;height: 8%;color: #ff86868a;font-size: 25px;justify-content: center;position: absolute;">
<text style="top: 10px;">
实时聊天室
</text>
</div>
<list style="height: 80%;">
<list-item for="{{message}}" class="{{$item.postId==id?'listItemRight':'listItemLeft'}}" >
<div class="listItemDiv" >
<text style="padding:5px;border-radius: 10px;font-size: 20px;margin: 5px;max-width: 70%;">
{{$item.message}}
</text>
</div>
</list-item>
</list>
<div style="position: absolute;left:10px;bottom: 20px;">
<textarea id="textarea" class="textarea" extend="true"
placeholder="请输入聊天信息"
onchange="inputChange" >
</textarea>
<button style="width: 75px;height: 50px;margin-left: 10px;background-color: #ff4848f5;" onclick="sendMessage"> 发送 </button>
</div>
</div>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
.container {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
left: 0px;
top: 0px;
width: 100%;
height: 100%;
}
.textarea {
placeholder-color: gray;
width: 70%;
}
.listItemDiv{
background-color: #ff87f3d0;
border-radius: 10px;
}
.listItemLeft{
margin: 10px;
width: 100%;
justify-content: flex-start;
}
.listItemRight{
margin: 10px;
width: 100%;
justify-content: flex-end;
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
Attachments related to the article can be downloaded by clicking the link below:
https://ost.51cto.com/resource/2210.
To learn more about open source, please visit :
51CTO Open Source Basic Software Community
https://ost.51cto.com .