Whose encryption key is written dead in the code?
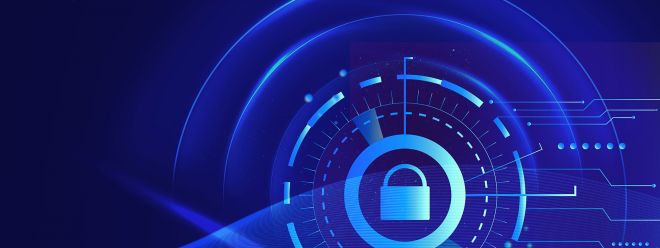
System design, protocol first.
Most people do not understand the design details of the protocol, and more use existing protocols for application layer design, such as:
- Use HTTP, design get/post/cookie parameters, and json package format;
- Use dubbo without delving into the details of the internal binary header and body;
In any case, knowing the principles of protocol design can be very helpful for a deep understanding of system communication.
First, the layered design of the protocol
The so-called "agreement" refers to the rules that both parties abide by, for example: divorce agreement, armistice agreement. The protocol has three elements: syntax, semantics, and timing:
- Syntax, i.e. the structure or format of data and control information;
- Semantics, that is, what kind of control information needs to be sent, what kind of action to complete, and what kind of response to make;
- Timing, that is, a detailed description of the order in which events are implemented;
Voice-over: The following text mainly focuses on grammar design.
Protocol design is usually divided into three layers: application layer protocol, security layer protocol, and transport layer protocol.
Let's take a look at how these three-layer protocols should be selected.
2. Application layer protocol design
There are three common types of application layer protocols: text protocol, binary protocol, and streaming XML protocol.
(1) Text Agreement
A text protocol refers to a communication transmission protocol that is "close to human written language". A typical protocol is the HTTP protocol. An example of a request message of the HTTP protocol is as follows:
GET / HTTP/1.1
User-Agent: curl
Host: musicml.net
Accept: */*
- 1.
- 2.
- 3.
- 4.
The characteristics of the text protocol are:
- Good readability and easy to debug;
- It has good scalability and can be extended through key:value;
- The parsing efficiency is not high, read line by line, split according to the colon, and parse the key and value;
- Not friendly to binary, such as voice/video, etc.;
(2) Binary protocol
The binary protocol is the binary protocol, which is typically the IP protocol. The following is an illustration of the IP protocol:
The binary protocol generally includes: generally includes:
- Fixed length header;
- Scalable variable-length package body;
- Generally, each field has a fixed meaning. Taking the IP protocol as an example, the first 4 bits represent the protocol version number (Version);
The characteristics of the binary protocol are:
- Poor readability and difficult to debug; Voiceover: Logging generally requires a toString() function to enhance readability.
- The extensibility is not good. If you want to extend the field, the old version of the protocol is not compatible, so there is usually a Version field in the design;
- The parsing efficiency is very high, there is almost no parsing cost, and each field of the binary stream represents a fixed meaning;
- Natural support for binary streams, such as voice/video;
Here is an example of a typical 16-byte binary fixed-length header:
//sizeof(cs_header)=16
struct cs_header {
uint32_t version;
uint32_t magic_num;
uint32_t cmd;
uint32_t len;
uint8_t data[];
}__attribute__((packed));
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
in:
(1) The first 4 bytes represent the version number;
(2) The next 4 bytes represent the magic number magic_num, which is used to solve the problem of data misalignment or packet loss;
Voice-over: For example, it is agreed that the magic number is 0x01020304. The received message, if the magic number matches, is considered to be a normal message, otherwise it is considered that the message is abnormal and the connection is disconnected.
(3) The next 4 bytes represent the command number command, and different command numbers correspond to different variable-length packet bodies;
(4) The last 4 bytes represent the length of the packet body to determine how many bytes the variable-length packet body has;
Here is an actual binary variable-length packet body:
message CUserLoginReq {
optional string username = 1;
optional string passwd = 2;
}
message CUserLoginResp {
optional uint64 uid =1;
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
It uses Google's Protobuf protocol, which is easy to see:
- The user name and password are passed in the request message;
- The response packet returns the user's uid;
PB is a very popular binary variable-length packet body protocol, and its advantages are:
- Universal, can generate C++, Java, PHP and other multi-language code;
- Built-in compression function;
- binary friendly;
- It has been widely used in the industry; voiceover: produced by Google, it must be a boutique.
Streaming XML Protocol Streaming XML seems to be a special case of textual protocols, and can also be considered a separate class. For example: xmpp is a typical streaming XML protocol, the following is a typical message of the xmpp protocol:
<message
to=’romeo@example.net’
from=’juliet@example.com’
type=’chat’
xml : lang=’en’>
<body>Wherefore art thou, Romeo?</body>
</message>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
It can be roughly judged from the xml tag that this is a chat message sent by romeo to juliet.
The XML protocol has several characteristics:
- Good readability and good expansibility, which are the characteristics of XML;
- The parsing cost is very high, and DOM tree analysis is required;
- The effective data transmission rate is ultra-low, and there are a large number of tags;
- Not friendly to binary, such as voice/video, etc.;
3. Security Layer Protocol Design
Security layer protocol design, in addition to using SSL, if you implement it yourself, there are the following three common solutions.
Voiceover: SSL key management is a problem.
(1) Fixed key
The server and the client agree on a key and an encryption algorithm (for example: AES). Each time the client sends a message, it uses the agreed algorithm and the agreed key to encrypt and then transmit it. After receiving the message, the end uses the agreed algorithm and the agreed key to decrypt it.
Voiceover: The security is low, and the security is based on the professional ethics of the programmer.
(2) One person, one secret
In simple terms, a person's key is fixed, but it varies from person to person. Common implementations are:
- Fixed encryption algorithm;
- The encryption key uses "a special attribute of the user", such as user uid, mobile phone number, QQ number, user password, etc.;
(3) One-time pad
That is, dynamic keys, one session and one key are more secure, and the key is negotiated before each session. The key negotiation process needs to go through two random generation of asymmetric keys and one random generation of symmetric encryption keys. The specific details are not expanded here.
Fourth, the transport layer protocol design
The optional protocols are TCP and UDP. Now TCP is basically used. With epoll and other technologies, multiple connections will not be a bottleneck. There is no problem with hundreds of thousands of links on a single machine.