How Does Vue Recognize the Text in the Picture and Convert it into Texts
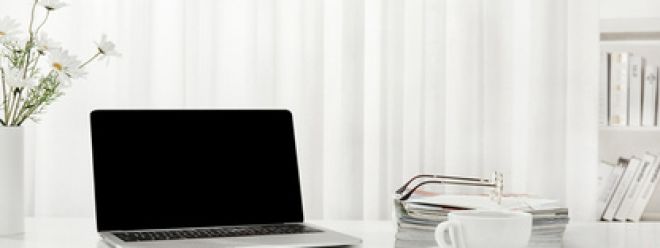
1. How does Vue recognize the text in the picture and convert it into text
In Vue.js, text recognition (OCR - Optical Character Recognition) in images usually requires the help of external libraries or services.
Vue.js itself is a JavaScript framework for building user interfaces and does not directly support OCR functionality.
However, you can integrate third-party OCR solutions such as Tesseract.js or use API services such as Google Cloud Vision API.
1.1. Using Tesseract.js
Tesseract.js is a JavaScript library based on the Tesseract OCR engine that allows you to recognize text in images on the front end.
Tesseract OCR is a very popular optical character recognition tool originally developed by HP and later maintained and developed by Google.
Tesseract.js encapsulates this powerful OCR engine into a form that can run in a browser environment, allowing web applications to directly use OCR technology without relying on server-side processing.
1.1.1. Install Tesseract.js
First, you need to add Tesseract.js to your project. If you are using npm and Node.js, you can install it via npm:
1.npm install tesseract.js
Using Tesseract.js
The following is an example of how to use Tesseract.js for image recognition in a Vue.js project:
- Import Tesseract.js :
Import Tesseract.js in your Vue component.
1.import Tesseract from 'tesseract.js';
- Create a method to recognize text in an image :
Create a method to handle image upload and use Tesseract.js to recognize text in the image.
1.export default {
2. data() {
3. return {
4. imageSrc: '', // 图片路径
5. textResult: '' // 识别后的文字结果
6. };
7. },
8. methods: {
9. async recognizeText() {
10. try {
11. const result = await Tesseract.recognize(
12. this.imageSrc, // 图像源
13. 'eng', // 语言模型,这里使用英语
14. {
15. logger: (m) => {
16. if (m.status === 'recognizing text') {
17. console.log(m);
18. }
19. }
20. }
21. );
22. this.textResult = result.data.text;
23. } catch (error) {
24. console.error('Error during OCR:', error);
25. }
26. },
27. handleImageUpload(event) {
28. const file = event.target.files[0];
29. if (!file) return;
30. const reader = new FileReader();
31. reader.onload = (e) => {
32. this.imageSrc = e.target.result;
33. this.recognizeText(); // 上传后立即识别
34. };
35. reader.readAsDataURL(file);
36. }
37. }
38.};
- Add a file input control in the template :
Add a file input box in the Vue template so that users can select an image to upload.
1.<template>
2. <div>
3. <input type="file" @change="handleImageUpload" accept="image/*">
4. <p v-if="textResult">{{ textResult }}</p>
5. </div>
6.</template>
In this way, when a user uploads a picture, Tesseract.js will recognize the text in it and display the results on the page.
Please note that the accuracy of OCR depends on many factors, such as image quality, text clarity, and the language model used. In addition, the recognition process may be time-consuming, especially for large images or complex text formats.
1.2. Using Google Cloud Vision API
If you choose to use the Google Cloud Vision API for OCR, you need to have a valid Google Cloud account and enable the Vision API. You can then process text recognition in images by sending requests to the API.
1.2.1. Setting up Google Cloud API:
Obtain an API key and configure your application to use the API.
1.2.2. Sending request to API:
Use Axios or Fetch API to send image data to Google Cloud Vision API and parse the returned results.
Since network requests are involved, this approach may increase the complexity of the application, including error handling, API call frequency limits, etc.
Please make sure to follow the license agreement when using any third-party libraries or services, and pay attention to data security and privacy protection.
The above is a method to recognize text in images in Vue.js.
The specific implementation may also require adjusting the code logic according to your actual needs.