An article to help you understand JavaScript While loop
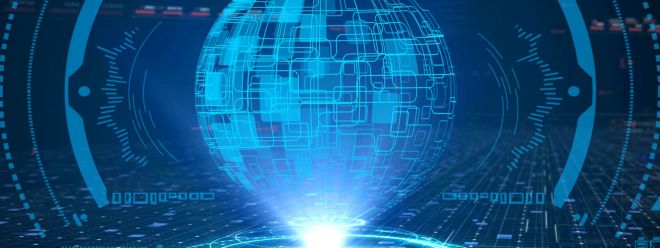
1. While loop
while loop executes the specified statements as long as the specified condition evaluates to true.
Syntax of while loop:
while (condition) {
statement
// 只要条件为真,就执行代码
}
- 1.
- 2.
- 3.
- 4.
example:
<!DOCTYPE html>
<html>
<title>项目</title>
<body>
<h1>JavaScript while 语句</h1>
<p>只要n小于5,就遍历一段代码:</p>
<script>
var n = 0;
while (n < 5) {
document.write("<br>The number is " + n);
n++;
}
</script>
</body>
</html>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
First, a variable is set before the loop begins (var n = 0;).
Then, define the condition under which the loop will run. As long as the variable is less than 5, the loop will continue, and each time the loop executes, the variable will increase by one (n++) Once the variable is not less than 5, the condition is false and the loop will end.
Notice:
If you want to use a variable with a condition, initialize it before the loop and then increment it inside the loop. If you forget to increment the variable, the loop will never end. This will crash your browser.
2. Infinite loop
An infinite loop, as the name suggests, is a loop that will keep running forever.
If you accidentally create an infinite loop, it can crash your browser or computer. It's important to be aware of infinite loops so that you can avoid them.
When the condition of the while statement is evaluated to true, a common infinite loop occurs.
while (true) {
statement
// 永远执行代码
}
- 1.
- 2.
- 3.
- 4.
An infinite loop will run forever, but the program can be terminated using the break keyword.
3. Do ... While Loop
The do...while loop is another form of the while loop. This loop will execute the code block once before checking if the condition is true, and then it will repeat the loop as long as the condition is true.
The syntax of the do...while loop is as follows:
do {
statement
// 要执行的语句
}
while (condition);
- 1.
- 2.
- 3.
- 4.
- 5.
example
<!DOCTYPE html>
<html>
<title>项目</title>
<body>
<h1>JavaScript do...while</h1>
<p>只要n小于5,就遍历一段代码:</p>
<script>
var n = 0;
do {
document.write("<br>数字: " + n);
n++;
}
while (n < 5);
</script>
</body>
</html>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
Don't forget to increment the variable used in the condition, otherwise the loop will never end.
Note: This loop will always execute at least once, even if the condition is false, because the code block is executed before the condition is tested.
example
var n = 5;
do {
document.write("<br>数字是 " + n);
n++;
}
while (n < 3); // false
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
4. Cycle Comparison
1.Difference between while and do...while loops
The while loop differs from the do…while loop in an important way. The while loop tests the condition to be evaluated at the beginning of each loop iteration, so if the conditional expression evaluates to false, the loop will never be executed.
On the other hand, with a do...while loop, the loop will always execute once even if the conditional expression evaluates to false, because unlike a while loop, the condition is evaluated at the end of the loop execution, not at the beginning. (Refer to Baidu).
2. For and While loops
The for loop uses a loop to get the fruit names from the fruits array.
example:
var fruits = ['strawberry', 'Mango', 'Banana', 'pineapple'];
var txt = '';
for (var i = 0; i < fruits.length; i++) {
txt += fruits[i] + '<br>';
}
- 1.
- 2.
- 3.
- 4.
- 5.
The while loop uses a loop to get the fruit names from the fruits array.
Example:
var fruits = ['strawberry', 'Mango', 'Banana', 'pineapple'];
var txt = '';
var i = 0;
while (i < fruits.length) {
txt += fruits[i] + '<br>';
i++;
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
You will find that the while loop is very similar to the for loop, except that the initialization and final expressions are omitted.
V. Conclusion
This article is based on JavaScript basics. It mainly introduces the basic applications of While loops, as well as another type of do ... while loops. It also introduces the differences between while and do ... while loops. It also explains in detail the advantages and disadvantages of For and While loops.
The code is very simple. I hope it can help you learn better.