Master Python's core tools: comprehensive analysis of list derivation, dictionary derivation and set derivation
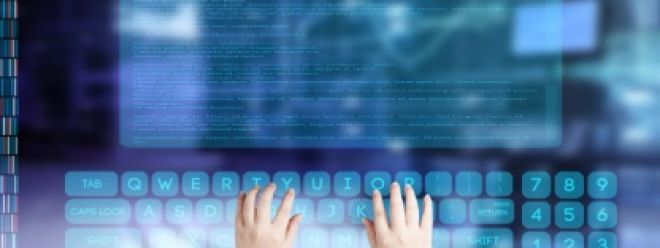
List comprehensions: the magic of building lists concisely
List comprehension is the most intuitive and efficient way to construct lists in Python. It allows you to complete loops, conditional judgments, and the generation of new elements in one line of code.
Basic Example: Square Number Generation
squares = [x**2 for x in range(1, 6)]
print(squares) # 输出:[1, 4, 9, 16, 25]
- 1.
- 2.
Condition filtering: filter even numbers
even_numbers = [x for x in range(10) if x % 2 == 0]
print(even_numbers) # 输出:[0, 2, 4, 6, 8]
- 1.
- 2.
Nested loops: matrix transpose
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
transposed = [[row[i] for row in matrix] for i in range(len(matrix[0]))]
print(transposed) # 输出:[[1, 4, 7], [2, 5, 8], [3, 6, 9]]
- 1.
- 2.
- 3.
Dictionary comprehensions: the art of building mappings
Dictionary comprehensions allow you to quickly create dictionaries where each key-value pair is evaluated from an expression.
Basic Example: Letter Counting
word = "comprehension"
char_count = {char: word.count(char) for char in set(word)}
print(char_count) # 输出:{'c': 1, 'o': 2, 'm': 1, 'p': 1, 'r': 2, 'e': 2, 'h': 1, 'n': 1, 's': 1, 'i': 1, 't': 1}
- 1.
- 2.
- 3.
Condition filtering: Age category
people = [{"name": "Alice", "age": 30}, {"name": "Bob", "age": 25}, {"name": "Charlie", "age": 35}]
age_groups = {person["name"]: "adult" if person["age"] >= 18 else "minor" for person in people}
print(age_groups) # 输出:{'Alice': 'adult', 'Bob': 'adult', 'Charlie': 'adult'}
- 1.
- 2.
- 3.
Set derivation: deduplication and set operations
Set comprehensions provide a convenient way to create sets and are particularly good at removing duplicates and performing operations between sets.
Remove duplicates and square
numbers = [1, 2, 2, 3, 4, 4, 5]
unique_squares = {x**2 for x in numbers}
print(unique_squares) # 输出:{1, 4, 9, 16, 25}
- 1.
- 2.
- 3.
Intersection and Square
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
common_squares = {x**2 for x in set1 & set2}
print(common_squares) # 输出:{9, 16}
- 1.
- 2.
- 3.
- 4.
Advanced Techniques: Nested and Chained Derivations
Derivations can be nested within each other and even combined with conditional expressions to implement complex logic.
Nested List Comprehensions: Matrix Multiplication
matrix_a = [[1, 2], [3, 4]]
matrix_b = [[5, 6], [7, 8]]
result = [[sum(a*b for a, b in zip(row_a, col_b)) for col_b in zip(*matrix_b)] for row_a in matrix_a]
print(result) # 输出:[[19, 22], [43, 50]]
- 1.
- 2.
- 3.
- 4.
Chaining derivations: complex transformations
data = [("apple", 2), ("banana", 4), ("cherry", 1)]
fruits_sorted_by_count = sorted(
(fruit for fruit, count in data),
key=lambda pair: pair[1],
reverse=True
)
print(fruits_sorted_by_count) # 输出:['banana', 'apple', 'cherry']
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
Conclusion
List derivation, dictionary derivation and set derivation are essential parts of Python. They greatly improve coding efficiency and code readability with concise and powerful expressions. Through the above examples, I hope to help you deeply understand the application of these three derivation methods and make them your right-hand man in your programming journey. By constantly exploring in daily programming practice, you will find more clever usage scenarios and further improve the performance and beauty of the code.