新手使用ABP 框架及注意事項:純後端視角
2024.06.19
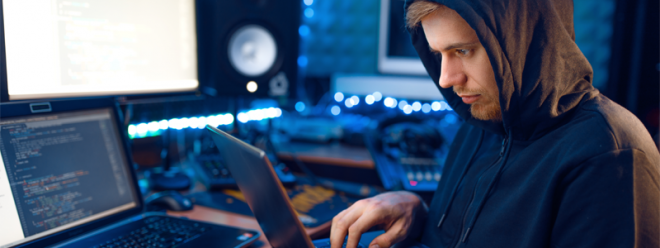
ABP(ASP.NET Boilerplate)框架是一個用於建立模組化、多租用戶應用程式的開源框架。它提供了一套完整的開發基礎設施,包括領域驅動設計(DDD)的許多最佳實踐、模組化設計、多租戶支援、身份驗證與授權、異常處理、日誌記錄等。對於新手來說,ABP框架可以大幅加速開發流程,但同時也需要注意一些關鍵事項以確保專案的順利進行。
一、ABP框架簡介
ABP架構基於.NET Core和Entity Framework Core,它遵循領域驅動設計(DDD)的原則,並提供了豐富的功能來幫助開發者快速建立企業級應用。透過使用ABP框架,開發者可以更專注於業務邏輯的實現,而無需過度關心底層技術細節。
二、新手使用ABP框架的注意事項
- 學習領域驅動設計(DDD):ABP框架是基於DDD建構的,因此理解DDD的基本概念(如聚合、實體、值物件、領域服務等)對於有效使用ABP至關重要。
- 模組化設計:ABP支援模組化開發,每個模組都有自己的功能和服務。新手應充分利用此特性,將應用程式拆分為多個模組,以提高程式碼的可維護性和可擴展性。
- 異常處理與日誌記錄:ABP提供了強大的異常處理和日誌記錄機制。確保在程式碼中妥善處理異常,並記錄必要的日誌訊息,以便於調試和故障排查。
- 身份驗證與授權:ABP整合了身份驗證和授權機制。合理配置和使用這些功能可以確保應用程式的安全性。
- 效能優化:雖然ABP框架本身已經進行了許多效能最佳化,但在實際開發中仍需要注意避免N+1查詢問題、合理使用快取等效能相關的最佳實務。
三、範例程式碼
以下是一個簡單的ABP框架使用範例,展示如何建立一個簡單的領域實體和服務。
1. 定義領域實體
首先,我們定義一個簡單的Product實體:
using Abp.Domain.Entities;
using Abp.Domain.Entities.Auditing;
public class Product : Entity<long>, IHasCreationTime
{
public string Name { get; set; }
public decimal Price { get; set; }
public DateTime CreationTime { get; set; }
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
2. 創建領域服務
接下來,我們建立一個簡單的領域服務來處理Product實體的業務邏輯:
using Abp.Domain.Services;
using System.Collections.Generic;
using System.Linq;
public class ProductManager : DomainService
{
private readonly IRepository<Product, long> _productRepository;
public ProductManager(IRepository<Product, long> productRepository)
{
_productRepository = productRepository;
}
public virtual void CreateProduct(string name, decimal price)
{
var product = new Product
{
Name = name,
Price = price,
CreationTime = Clock.Now // 使用ABP提供的Clock服务获取当前时间
};
_productRepository.Insert(product);
}
public virtual List<Product> GetAllProducts()
{
return _productRepository.GetAllList();
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
3. 使用領域服務
在應用服務層,你可以呼叫ProductManager來處理業務邏輯:
public class ProductAppService : ApplicationService, IProductAppService
{
private readonly ProductManager _productManager;
public ProductAppService(ProductManager productManager)
{
_productManager = productManager;
}
public void Create(CreateProductInput input)
{
_productManager.CreateProduct(input.Name, input.Price);
}
public List<ProductDto> GetAll()
{
var products = _productManager.GetAllProducts();
return ObjectMapper.Map<List<ProductDto>>(products); // 使用ABP的ObjectMapper进行DTO映射
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
在這個例子中,我們展示瞭如何在ABP框架中定義領域實體、建立領域服務,並在應用程式服務層中使用這些服務。請注意,為了簡化範例,我們省略了一些ABP框架的特性和最佳實踐,例如依賴注入、驗證、權限檢查等。在實際專案中,你應根據具體需求來完善這些面向。
四、總結
ABP框架為開發者提供了一個強大的基礎設施來建立模組化、可擴展的應用程式。作為新手,掌握DDD的基本原則、模組化設計、異常處理與日誌記錄等關鍵概念對於成功使用ABP至關重要。透過不斷學習和實踐,你將能夠充分利用ABP框架的優勢,快速建立高品質的企業級應用。