C++ useful encryption library: Crypto++
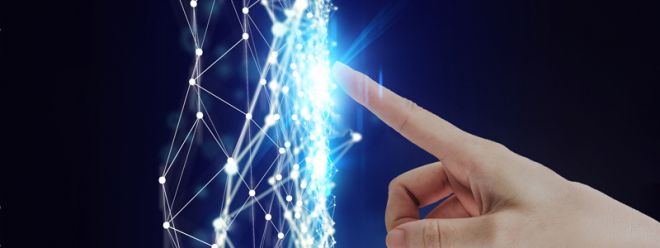
In many application scenarios, cryptographic algorithms need to be used to protect the security and integrity of data.
The Crypto++ library provides a convenient way to use these algorithms and is a widely used open source cryptography library.
Crypto++
Crypto++ is an open source encryption library for cryptographic operations such as encryption, decryption, hashing, signing, and verification.
Crypto++ is a C++ library that provides a wealth of cryptographic algorithms, such as public key encryption (RSA, DSA), symmetric encryption (AES, DES, RC6), hash functions (SHA-1, SHA-2), and message authentication codes. (HMAC), etc., supporting various application scenarios.
Crypto++ provides a rich set of cryptography algorithms, including many encryption algorithms and protocols commonly used in cryptography.
Basic features:
- Supports multiple encryption algorithms: Crypto++ supports multiple symmetric encryption algorithms (such as AES, DES, 3DES, etc.) and public key encryption algorithms (such as RSA, ECC, etc.).
- Easy to use: Crypto++ provides a simple API, making cryptographic operations easy to understand and implement.
- Platform independent: The Crypto++ library can run on various platforms, including Windows, Linux, macOS, etc.
- Safe and reliable: Crypto++ uses widely proven cryptographic algorithms and modes to ensure data security and integrity.
Crypto++ compilation
1 Overview
Crypto++ source code download address: https://github.com/weidai11/cryptopp
2. Open the Visual Studio project
Visual Studio opens the sln project file in the directory:
Select the cryptlib project and compile its static library:
Modify Windows SDK version:
Modify the runtime library to MD:
Compile the Debug version first:
Continue to compile the Release version:
The compilation results are in the current Output directory:
3. Organize library files
For ease of use, you can organize related files into a folder:
Among them, copy all header files in the source directory in include:
Crypto++ library usage
1.Project settings
(1) Header file reference
Add header file reference in project settings:
(2) Library file reference
Add library file search path in project settings:
Add the library files to be referenced:
2. Code examples
Here is a simple example of AES encryption and decryption using the Crypto++ library:
#include <iostream>
#include <string>
#include <aes.h>
#include <modes.h>
#include <filters.h>
int main()
{
using namespace CryptoPP;
// 明文和密钥
std::string plainText = "Hello, Crypto++!";
std::string keyStr = "0123456789abcdef"; // 设置密钥字符串
byte key[AES::MAX_KEYLENGTH];
memset(key, 0x00, AES::MAX_KEYLENGTH);
size_t keyLen = keyStr.length() < AES::MAX_KEYLENGTH ? keyStr.length() : AES::MAX_KEYLENGTH;
memcpy(key, keyStr.c_str(), keyLen);
// 加密过程
byte iv[AES::BLOCKSIZE];
memset(iv, 0x00, AES::BLOCKSIZE);
std::string cipherText;
{
CFB_Mode<AES>::Encryption encr;
encr.SetKeyWithIV(key, AES::MAX_KEYLENGTH, iv);
StringSource(plainText, true, new StreamTransformationFilter(encr, new StringSink(cipherText)));
}
// 打印密文
std::cout << "密文: " << std::string(cipherText.begin(), cipherText.end()) << std::endl;
// 解密过程
std::string recoveredText;
{
CFB_Mode<AES>::Decryption decr;
decr.SetKeyWithIV(key, AES::MAX_KEYLENGTH, iv);
StringSource(cipherText, true, new StreamTransformationFilter(decr, new StringSink(recoveredText)));
}
// 打印解密后的明文
std::cout << "明文: " << recoveredText << std::endl;
return 0;
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
The running results are as follows: