A useful Python library: pretty-errors makes your bugs look different
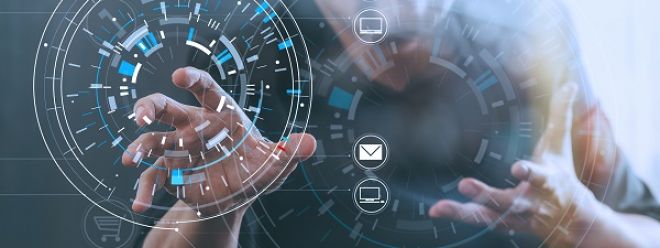
Python is a high-level programming language. Its ease of learning and powerful functions make it the language of choice for many developers. However, when we write Python code, sometimes we encounter some errors that may take us a long time to debug and solve. To solve this problem, there is a Python library called PrettyErrors that helps us better understand and debug errors in Python code.
PrettyErrors is a Python library that converts Python error messages into a more understandable format and prints them on the terminal. Using PrettyErrors allows us to identify and resolve errors in our code faster, thereby improving our development efficiency.
Here's how to use the PrettyErrors library:
1. Install PrettyErrors library
To use the PrettyErrors library, we first need to install it. The PrettyErrors library can be installed in the terminal using the pip command:
```
pip install pretty_errors
```
- 1.
- 2.
- 3.
- 4.
- 5.
2. Import PrettyErrors library
In Python code, we need to import the PrettyErrors library to use it. The PrettyErrors library can be imported using the following code:
```
import pretty_errors
```
- 1.
- 2.
- 3.
- 4.
- 5.
3. Configure the PrettyErrors library
After importing the PrettyErrors library, we need to configure it for use. The PrettyErrors library can be configured using the following code:
```
pretty_errors.configure(
separator_character = '*',
filename_display = pretty_errors.FILENAME_EXTENDED,
line_number_first = True,
display_link = True,
lines_before = 5,
lines_after = 2,
line_color = pretty_errors.RED + '> ' + pretty_errors.BOLD,
code_color = ' ' + pretty_errors.BLUE,
truncate_code = True,
display_locals = True
)
```
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
In the above code, we can see some configuration options, such as delimiter character, file name display method, whether to display links, display the number of lines before and after the error line, line number and code color, etc. Depending on your needs, you can customize these options to fit your code.
4. Run Python code
After completing the configuration of the PrettyErrors library, we can run the Python code and wait for errors to occur. When an error occurs, the PrettyErrors library converts the error message into an easy-to-understand format and prints it on the terminal.
For example, Python throws a NameError when we use undefined variables in our code. Without using the PrettyErrors library, Python will print a simple error message as follows:
```
NameError: name 'x' is not defined
```
- 1.
- 2.
- 3.
- 4.
- 5.
However, when we use the PrettyErrors library, Python converts the error message into a more understandable format and prints it on the terminal, as shown below:
```
************************* NameError *************************
name 'x' is not defined
-------------------------------------------------------------
Traceback (most recent call last):
File "example.py", line 3, in <module>
print(x)
File "/usr/local/lib/python3.9/site-packages/pretty_errors/__init__.py", line 324, in _pretty_error
code_lines, offending_line_index = _find_offending_line(lines, line_number)
File "/usr/local/lib/python3.9/site-packages/pretty_errors/__init__.py", line 248, in _find_offending_line
raise ValueError("Line number out of range")
ValueError: Line number out of range
```
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
In the above error message, we can see more detailed error information, such as error type, error message, file name and line number where the error occurred, etc. This information helps us identify and resolve errors in our code more quickly.
Summarize
PrettyErrors is a very useful Python library that helps us better understand and debug errors in Python code. Use PrettyErrors to convert Python error messages into an easy-to-understand format and print them on the terminal. By configuring the PrettyErrors library, we can customize the format of error messages to suit our code. In actual development, using PrettyErrors can improve our development efficiency and reduce debugging time.