Data analysis tool: Application skills of Python counter Counter
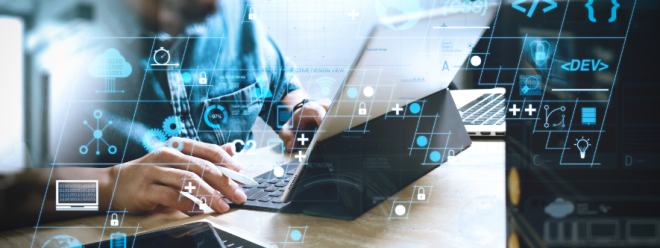
In Python, writing code that is readable and Pythonic is crucial. Refactoring techniques refer to adjusting the code structure and style to make it more consistent with Python conventions and standards, thereby improving the readability, simplicity and maintainability of the code. This article takes an in-depth look at eight refactoring techniques to help you write more Pythonic code.
1. Use generator expressions to replace list comprehensions
List comprehensions are very useful when creating lists, but they can take up a lot of memory when the amount of data is large. Generator expressions use lazy evaluation and will not generate all elements at once.
# 列表推导式
list_comp = [x * 2 for x in range(10)]
# 生成器表达式
gen_exp = (x * 2 for x in range(10))
- 1.
- 2.
- 3.
- 4.
- 5.
2. Use generator functions to optimize the iterative process
The generator function generates iterators through the yield statement, effectively improving the readability and efficiency of the code.
# 生成器函数
def countdown(num):
while num > 0:
yield num
num -= 1
- 1.
- 2.
- 3.
- 4.
- 5.
3. Use decorators to simplify repetitive tasks
Decorators are powerful tools in Python for modifying function behavior, such as logging, performance measurement, and permission checking.
# 装饰器示例
def my_decorator(func):
def wrapper(*args, **kwargs):
print("Before function execution")
result = func(*args, **kwargs)
print("After function execution")
return result
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
4. Use built-in functions and methods to simplify code
Python's built-in functions and methods provide many convenient operations, such as enumerate(), zip(), sorted(), etc.
# 使用enumerate()简化代码
my_list = ['apple', 'banana', 'orange']
for index, value in enumerate(my_list):
print(index, value)
- 1.
- 2.
- 3.
- 4.
5. Optimize conditional expressions
Simplifying conditionals and using Boolean operators can make code more compact and readable.
# 简化条件表达式
x = 10
result = "Even" if x % 2 == 0 else "Odd"
print(result)
- 1.
- 2.
- 3.
- 4.
6. Parameterization and deconstruction of functions
Using features such as *args and **kwargs parameters, tuple destructuring, and dictionary destructuring, you can handle function parameter passing more flexibly.
# 使用*args和**kwargs
def my_func(*args, **kwargs):
for arg in args:
print(arg)
for key, value in kwargs.items():
print(f"{key}: {value}")
my_func(1, 2, 3, name='Alice', age=30)
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
7. Refactor object-oriented programming
Optimization of object-oriented programming includes rational use of inheritance, avoiding multiple inheritance, using properties instead of directly exposing properties, etc.
# 使用特性(property)
class Circle:
def __init__(self, radius):
self._radius = radius
@property
def radius(self):
return self._radius
@radius.setter
def radius(self, value):
if value < 0:
raise ValueError("Radius cannot be negative")
else:
self._radius = value
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
Summarize
The application of refactoring techniques can make Python code clearer, more concise, and easier to maintain. Through the rational use of generators, decorators, built-in functions, optimized conditional expressions, etc., the Pythonic level of the code can be greatly improved. These techniques not only help improve code quality, but also improve team collaboration efficiency and are of great benefit in long-term maintenance.
These refactoring techniques are designed to help developers make better use of Python's features and syntax and write more expressive and readable code. Deeply understanding and applying these techniques will make your code more Pythonic, easier to understand and maintain.