An in-depth analysis of the causes of deadlock in C++
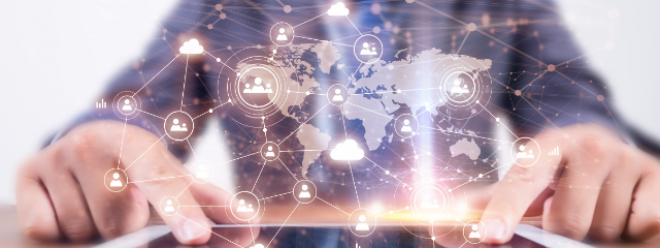
In concurrent programming, deadlock is a vexing problem that not only causes the program to stall, but is often difficult to debug and fix. This article will delve into the main causes of deadlocks in C++ concurrent programming, and help readers better understand this concept through a combination of code examples and text explanations.
1. Competition conditions and resource sharing
In a multi-threaded environment, race conditions occur when multiple threads access and modify shared resources at the same time. If this access is not properly synchronized, it may lead to data inconsistency or even deadlock.
For example, consider a simple bank account transfer scenario. The two threads represent the transfer operations of two users respectively. If two threads read the balance of the same account at the same time and update the balance at the same time after calculation, the final balance may be wrong.
// 假设这是一个全局的共享资源
int account_balance = 1000;
void transfer(int amount) {
// 读取余额
int bal = account_balance;
// 模拟一些其他操作
std::this_thread::sleep_for(std::chrono::milliseconds(10));
// 更新余额
account_balance = bal - amount; // 这里存在竞态条件
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
In the above code, if two threads call the transfer function almost at the same time, they may read the same balance and calculate and update it based on this balance, resulting in a balance error.
2. Improper lock use
Locks are a common mechanism used to synchronize access to shared resources. However, deadlocks can also result if locks are used improperly.
Nested locks: A deadlock occurs when one thread holds one lock while requesting another lock, and another thread, on the contrary, also holds the second lock and requests the first lock.
std::mutex mtx1, mtx2;
void thread1() {
mtx1.lock();
std::this_thread::sleep_for(std::chrono::milliseconds(10));
mtx2.lock(); // 如果此时mtx2被thread2持有,则会发生死锁
// ...
mtx2.unlock();
mtx1.unlock();
}
void thread2() {
mtx2.lock();
std::this_thread::sleep_for(std::chrono::milliseconds(10));
mtx1.lock(); // 如果此时mtx1被thread1持有,则会发生死锁
// ...
mtx1.unlock();
mtx2.unlock();
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- Inconsistent ordering of locks: Deadlocks can also result if different threads request locks in different orders.
- Forgetting to release a lock: If a thread acquires a lock but forgets to release it, other threads waiting for the lock will be blocked forever.
3. Misuse of condition variables
Condition variables are often used to synchronize state changes between multiple threads. However, if condition variables are used inappropriately, deadlocks can result.
For example, when condition variables are used in conjunction with locks, problems may occur if the wait() function is called in a thread without first acquiring the corresponding lock, or if the condition is not rechecked after calling wait().
std::mutex mtx;
std::condition_variable cv;
bool ready = false;
void waitThread() {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, []{return ready;}); // 等待条件满足
// ...
}
void signalThread() {
std::this_thread::sleep_for(std::chrono::milliseconds(10));
ready = true;
cv.notify_one(); // 通知等待线程
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
In the above code, the waitThread thread acquires the lock before waiting for the condition to be met. This is the correct way to use it because it ensures atomicity between wait() calls and condition checks.
4. Exhaustion of resources
In concurrent programming, resource exhaustion is another important cause of deadlock. This situation usually occurs when system resources are limited and the program's needs exceed what the system can provide. The following are some specific situations where resource exhaustion leads to deadlock:
- File descriptor exhaustion: Every time a process opens a file or socket in the operating system, it uses a file descriptor. If a program opens a large number of files or network connections without closing them, it may exhaust the number of file descriptors allocated to it by the system. When a program tries to open more files or sockets, it fails because it cannot obtain a new file descriptor, which can lead to a deadlock or program crash.
- Thread resource exhaustion: The operating system has certain limits on the number of threads running simultaneously. If a program creates too many threads without managing them appropriately (for example, by not terminating threads that are no longer needed in a timely manner), it can exhaust the system's thread resources. When the program tries to create more threads, it will be blocked because it cannot obtain new thread resources, which may also lead to deadlock or program crash.
- Memory resource exhaustion: If a program consumes a large amount of memory while running and does not release the no longer used memory space in time, the system's memory resources may be exhausted. When a program tries to allocate more memory, it fails because it cannot acquire new memory space, which can also lead to deadlock or program crash.
In order to avoid deadlock problems caused by resource exhaustion, programmers need to take some precautions:
- Release resources promptly: Make sure that after you have finished using resources such as files, sockets, threads, or memory, you close or release them promptly so that other programs or threads can use these resources.
- Resource limits: Set reasonable resource limits in the program to avoid requesting too many resources at one time.
- Error handling: When requesting resources, consider possible failures and write appropriate error handling code so that errors can be handled appropriately when resources are insufficient, rather than causing deadlocks.
By properly managing resources, programmers can reduce the risk of deadlock caused by resource exhaustion and improve the robustness and reliability of the program.
in conclusion
Deadlock is a complex problem in concurrent programming that can be caused by a variety of reasons. In order to avoid deadlock, programmers need to carefully design concurrency control strategies, ensure the correct use of locks and condition variables, and always pay attention to the usage of system resources. By deeply understanding and practicing these principles, we can write more robust and efficient concurrent programs.