JS little knowledge, how to convert CSV to JSON string
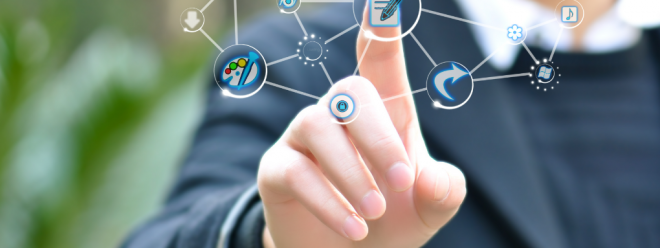
Use csvtojson third-party library
You can use the csvtojson library to quickly convert CSV to JSON string in JavaScript:
index.js
import csvToJson from 'csvtojson';
const csvFilePath = 'data.csv';
const json = await csvToJson().fromFile(csvFilePath);
console.log(json);
- 1.
- 2.
- 3.
- 4.
data.csv file
For example, the content of this data.csv file is as follows:
color,maxSpeed,age
"red",120,2
"blue",100,3
"green",130,2
- 1.
- 2.
- 3.
- 4.
The final generated JSON array string content is as follows:
[
{ color: 'red', maxSpeed: '120', age: '2' },
{ color: 'blue', maxSpeed: '100', age: '3' },
{ color: 'green', maxSpeed: '130', age: '2' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
Install csvtojson
Before using csvtojson, you need to install it into our project. You can do this using NPM or the Yarn CLI.
npm i csvtojson
# Yarn
yarn add csvtojson
- 1.
- 2.
- 3.
- 4.
Once installed, introduce it into your project as follows:
import csvToJson from 'csvtojson';
// CommonJS
const csvToJson = require('csvtojson');
- 1.
- 2.
- 3.
- 4.
Import CSV files through the fromFile() method
We call the default exported function of the csvtojson module to create the object that will convert the CSV. This object has a bunch of methods, each related to CSV to JSON conversion in some way, fromFile() being one of them.
It accepts the name of the CSV file to convert and returns a Promise since the conversion is an asynchronous process. The Promise will be parsed using the resulting JSON string.
Directly convert CSV string to JSON, fromString()
To convert directly from a CSV data string rather than a file, you can use the asynchronous fromString() method of the conversion object instead:
index.js
import csvToJson from 'csvtojson';
const csv = `"First Name","Last Name","Age"
"Russell","Castillo",23
"Christy","Harper",35
"Eleanor","Mark",26`;
const json = await csvToJson().fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
[
{ 'First Name': 'Russell', 'Last Name': 'Castillo', Age: '23' },
{ 'First Name': 'Christy', 'Last Name': 'Harper', Age: '35' },
{ 'First Name': 'Eleanor', 'Last Name': 'Mark', Age: '26' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
Custom CSV to JSON conversion
The default export function of csvtojson accepts an object that specifies options to customize the conversion process.
One of the options is header, which is an array specifying the headers in the CSV data, which can be replaced with a more readable alias.
index.js
import csvToJson from 'csvtojson';
const csv = `"First Name","Last Name","Age"
"Russell","Castillo",23
"Christy","Harper",35
"Eleanor","Mark",26`;
const json = await csvToJson({
headers: ['firstName', 'lastName', 'age'],
}).fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
[
{ firstName: 'Russell', lastName: 'Castillo', age: '23' },
{ firstName: 'Christy', lastName: 'Harper', age: '35' },
{ firstName: 'Eleanor', lastName: 'Mark', age: '26' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
The other is delimeter, which is used to represent the character that separates columns:
import csvToJson from 'csvtojson';
const csv = `"First Name"|"Last Name"|"Age"
"Russell"|"Castillo"|23
"Christy"|"Harper"|35
"Eleanor"|"Mark"|26`;
const json = await csvToJson({
headers: ['firstName', 'lastName', 'age'],
delimiter: '|',
}).fromString(csv);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
Output:
[
{ firstName: 'Russell', lastName: 'Castillo', age: '23' },
{ firstName: 'Christy', lastName: 'Harper', age: '35' },
{ firstName: 'Eleanor', lastName: 'Mark', age: '26' }
]
- 1.
- 2.
- 3.
- 4.
- 5.
We can also use the ignoreColumns attribute, an option that uses regular expressions to ignore certain columns.
import csvToJson from 'csvtojson';
const csv = `"First Name"|"Last Name"|"Age"
"Russell"|"Castillo"|23
"Christy"|"Harper"|35
"Eleanor"|"Mark"|26`;
const json = await csvToJson({
headers: ['firstName', 'lastName', 'age'],
delimiter: '|',
ignoreColumns: /lastName/,
}).fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
Convert CSV to row array
By setting the output option to "csv" we can generate a list of arrays, where each array represents a row and contains the values of all columns of that row.
As follows:
index.js
import csvToJson from 'csvtojson';
const csv = `color,maxSpeed,age
"red",120,2
"blue",100,3
"green",130,2`;
const json = await csvToJson({
output: 'csv',
}).fromString(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
[
[ 'red', '120', '2' ],
[ 'blue', '100', '3' ],
[ 'green', '130', '2' ]
]
- 1.
- 2.
- 3.
- 4.
- 5.
Use native JS to process CSV and convert to JSON
We can also convert CSV to JSON without using any third-party library.
index.js
function csvToJson(csv) {
// \n or \r\n depending on the EOL sequence
const lines = csv.split('\n');
const delimeter = ',';
const result = [];
const headers = lines[0].split(delimeter);
for (const line of lines) {
const obj = {};
const row = line.split(delimeter);
for (let i = 0; i < headers.length; i++) {
const header = headers[i];
obj[header] = row[i];
}
result.push(obj);
}
// Prettify output
return JSON.stringify(result, null, 2);
}
const csv = `color,maxSpeed,age
"red",120,2
"blue",100,3
"green",130,2`;
const json = csvToJson(csv);
console.log(json);
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
Output:
[
{
"color": "color",
"maxSpeed": "maxSpeed",
"age": "age"
},
{
"color": "\"red\"",
"maxSpeed": "120",
"age": "2"
},
{
"color": "\"blue\"",
"maxSpeed": "100",
"age": "3"
},
{
"color": "\"green\"",
"maxSpeed": "130",
"age": "2"
}
]
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
You can improve the above code to handle more complex CSV data.
Finish
That’s it for today’s sharing, how to convert CSV to JSON string, have you learned it?