Learn Python network programming from scratch: Explore the TCP protocol and practical demonstrations!
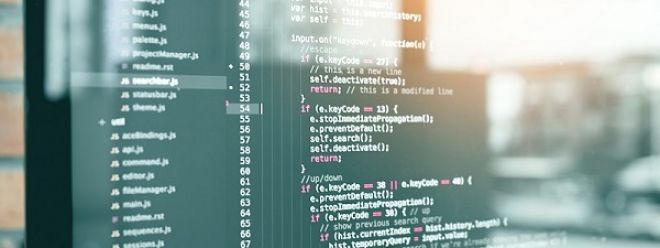
Learn Python network programming from scratch: Explore the TCP protocol and practical demonstrations!
Python is a high-level programming language with an extensive network programming library.
These libraries allow Python developers to communicate using TCP and other network protocols.
In this article, we'll explore the TCP protocol and use a simple example to demonstrate how to write network code in Python.
Introduction to TCP protocol
The TCP (Transmission Control Protocol) protocol is the basic transmission protocol for the Internet and many other networks.
The TCP protocol ensures reliable transmission of data over the network, including mechanisms to detect lost packets and request resends, as well as to deal with out-of-order arrival of packets and packet loss.
Therefore, TCP is a more reliable protocol compared to other protocols such as UDP, ICMP, and IP.
The TCP protocol is a connection-based protocol, so before data can be transmitted, a connection must be established.
Connecting involves creating and maintaining a virtual pipe (called a socket or endpoint) through which data can be transferred.
After the connection is established, data can be transferred between the two computers via pipes.
After the transfer is complete, the connection can be closed to release used resources and terminate the network connection.
TCP/IP model
The TCP protocol is based on the TCP/IP suite protocol stack.
In this protocol stack, each layer handles specific tasks and relies on the layers below to complete them.
The main layers of the TCP/IP model are:
- Application layer: This layer is the interface between applications and network protocols, including application protocols such as FTP, HTTP, and Telnet.
- Transport layer: This layer is the core of all data transmission, and protocols include TCP and UDP.
- Network layer: This layer uses IP protocol for packet routing.
- Link layer: This layer includes the physical layer and the data link layer. The task between these two layers is to establish and maintain data link connections on the physical network connection.
Establish TCP connection
To establish a TCP connection in Python, you need to use the socket library.
The socket library provides various socket functions and constants that make communication between Python and the network easier.
In order to establish a TCP connection, the following steps are required:
- create a socket
- bind socket
- Listening socket
- Accept connection requests from clients
Below, we will perform these steps in Python and create a simple server that listens for connection requests from clients.
import socket
# 创建一个 TCP/IP 套接字
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 绑定套接字到特定的地址和端口
server_address = ('localhost', 8888)
print('Starting up on {} port {}'.format(*server_address))
server_socket.bind(server_address)
# 开始监听连接
server_socket.listen(1)
# 等待连接请求
print('Waiting for a connection...')
connection, client_address = server_socket.accept()
print('Connection from', client_address)
# 处理请求
while True:
data = connection.recv(1024)
print('Received {!r}'.format(data))
if data:
connection.sendall(data)
else:
break
# 关闭连接
connection.close()
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
Let's explain line by line how this program works:
- First, we imported the socket library and created a TCP/IP socket. The socket.AF_INET parameter specifies that the socket will use an IPv4 cipher, and the socket.SOCK_STREAM parameter specifies that this is a stream socket.
- We then use the bind() function to bind the socket to a specific IP address and port. Here, we bind port 8888 on localhost.
- Next, we listen for connections. In this example, the socket is set to wait for at most 1 connection request.
- Finally, we use the accept() function to accept the connection request from the client. This function waits until a client connects. Once the connection is accepted, the accept() function returns a new socket and the client's address information.
- From here, we can handle the client's request. In this example, we simply read any data sent by the client and send them back to the client. When the client sends empty data, the loop terminates and the connection code closes.
Make a TCP connection
To connect to the Python server, we need to use another socket to represent the client endpoint.
The client socket needs to use the same protocol as the server socket.
The initialized IP address and port must be the same as the binding address and port used by the server.
The steps for client connection are as follows:
- create a socket
- Execute connection
Below, we will perform these steps in Python and create a simple client to connect to the server we created earlier.
import socket
# 创建一个 TCP/IP 套接字
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 连接到服务器
server_address = ('localhost', 8888)
print('Connecting to {} port {}'.format(*server_address))
client_socket.connect(server_address)
# 发送数据
message = b'This is a test message'
client_socket.sendall(message)
# 接收数据
data = client_socket.recv(1024)
print('Received', repr(data))
# 关闭连接
client_socket.close()
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
Let's explain line by line how this program works:
- First, we imported the socket library and created a TCP/IP socket using the same protocol as the server.
- Then, we use the connect() function to connect to the server. The connect() function requires specifying the IP address and port of the connection.
- Once we are connected to the server we can send data. In this example, we simply send a test message.
- Finally, we wait for the data sent back by the receiving server. Once the data is received we can output it and close the client socket.
in conclusion
This article provides a brief introduction to the TCP protocol and its use in Python programs.
Using the socket library, you can create sockets, bind sockets, listen to sockets, accept client connections, and send and receive data to other computers.
With these steps, we can connect the program to the TCP protocol and initiate network communication.
Of course, this article only introduces the basic principles and applications of the TCP protocol. There is still a lot of in-depth and complex knowledge that needs to be learned and mastered.