A complete guide to using Go language built-in packages!
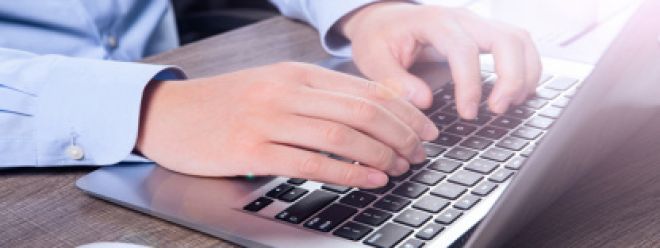
A complete guide to using Go language built-in packages!
Introduction to commonly used built-in packages in Go language
I. Overview
The Go language has built-in packages with many commonly used functions, which can be directly used for project development. Proficiency in built-in packages can greatly improve the efficiency of Go language programming.
This article will briefly introduce some commonly used built-in packages in the Go language, including
- fmt package: formatting and printing
- os package: platform related functions
- io package: input and output operations
- bufio package: cached IO
- net/http package: HTTP network communication
- encoding package: data encoding and decoding
- flag package: command line parameter parsing
- reflect package: reflection mechanism
- sort package: sorting functions
- testing package: testing framework
2. fmt package
The fmt package implements formatted IO related functions:
- Print series functions print output
- Scan series function format input
- Sprintf formatted string concatenation
- Errorf error creation
3. os package
The os package provides platform-dependent functions:
- File operations: open, delete, rename files
- Directory operations: create and delete directories
- Environment variables: getting and setting environment variables
- Process/command operations: start processes, etc.
Cross-platform code can be written using the os package.
4. io package
The io package provides basic IO primitives:
- Reader/Writer interface
- Pipe pipe communication
- EOF end flag
- LimitedReader reads according to restrictions
The io package defines an abstract interface for all IO operations.
5. bufio package
bufio implements buffered IO operations:
- Buffering reading and writing improves efficiency
- Read, Write series methods
- Custom buffer read and write objects
- Expandable buffering
Suitable for IO operation optimization.
6. net/http package
The net/http package provides HTTP client and server implementations:
- Client sends request
- Server handles the request
- Transport underlying transport
- response/request object
- timeout mechanism
Many web services are built on the net/http package.
7. encoding package
encoding implements various data encoding and decoding:
- JSON encoding and decoding
- XML encoding and decoding
- Base64 encoding and decoding
The encoding package can be used in daily encoding conversion scenarios.
8. flag package
The flag package implements command line parameter parsing:
- Define command line flag parameters
- Parse command line input parameters
- Commonly used flag types (bool, int, etc.)
- Custom flag type
Many CLI tools use the flag package.
9. reflect package
reflect implements the reflection mechanism:
- TypeOf reflection type information
- ValueOf reflection value information
- Setting variables via reflection
- Reflection calling method
Reflection is an important capability of dynamic languages.
10. sort package
The sort package implements various data sorting:
- Sort slices of built-in types
- Sort custom types
- Sort by multiple conditions
- Stability ranking
Just implement a few interfaces to sort.
11. Testing package
testing provides testing functions:
- Add test case
- Run tests and generate reports
- coverage calculation
- benchmark stress test
- mock data
Can write unit tests etc.
Summarize
Go language has many commonly used packages built-in, and rational use can greatly improve development efficiency. This article provides a brief overview of some of them, hoping to help you better use the built-in packages of the Go language.