Implement Http service in Qt to receive POST request
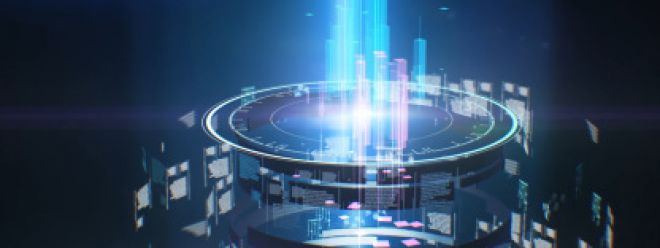
Implement Http service in Qt to receive POST request
Hi everyone! Make a simple record of the following knowledge points and share them with your friends!
First, let's understand a few concepts:
The difference between websocket server and http server
WebSocket server and HTTP server are two different types of servers, and they differ in terms of protocols, connection methods, and communication modes.
- Protocol: HTTP servers communicate using the HTTP protocol, while WebSocket servers use the WebSocket protocol. The HTTP protocol is stateless, the client initiates a request, and the server closes the connection immediately after responding to the request. The WebSocket protocol allows a persistent connection between a client and a server, two-way communication.
- Connection mode: The HTTP server adopts the "request-response" mode, that is, the client sends a request to the server, and the server disconnects after responding. Each request requires a connection re-establishment. After the initial handshake, the WebSocket server establishes a persistent connection, allowing two-way communication, and the client and server can send messages at any time.
- Communication mode: The HTTP server is based on the request-response mode, the client initiates a request, and the server responds. Each request and response is independent and has no persistence. The WebSocket server supports two-way communication. The client and server can interact in real time by sending messages, and the server can actively push messages to the client.
Generally speaking, the HTTP server is suitable for traditional client-server communication, and each request needs to re-establish the connection, which is suitable for request-response scenarios. WebSocket server is suitable for scenarios that require real-time two-way communication, suitable for chat applications, real-time data updates, etc.
It should be noted that the WebSocket protocol uses the HTTP protocol for an initial handshake when establishing a connection, so a WebSocket server can be implemented on an HTTP server. However, WebSocket servers offer more features and optimizations to support real-time communication needs.
Common HTTP request methods
In the HTTP protocol, common HTTP request methods (also known as HTTP verbs) include the following:
- GET: Used to obtain resources from the server, it specifies the URL of the resource to be obtained in the request. GET requests generally have no side effects on server data and are idempotent, i.e. multiple times of the same GET request should produce the same result.
- POST: Used to submit data to the server and request the server to process the data. The data of the POST request will be included in the message body of the request and used to create, update or delete resources. POST requests are generally not idempotent, that is, multiple identical POST requests may produce different results.
- PUT: It is used to upload data to the server and request the server to store it on the specified URL. A PUT request is similar to a POST request, but it is typically used to replace or update a resource.
- DELETE: Used to request the server to delete the specified resource.
- HEAD: Similar to a GET request, but the server only returns the response header information, not the actual resource content. HEAD requests are commonly used to get metadata about a resource or to check the existence and status of a resource.
- OPTIONS: Used to request the server to provide information about supported request methods, response headers, and other options.
- PATCH: It is used to partially update the resource, that is, only part of the content of the resource is modified.
In addition to the above common HTTP request methods, HTTP/1.1 also introduces some extended request methods, such as TRACE, CONNECT, PROPFIND, etc. These methods are less used in specific application scenarios.
In practical applications, developers choose appropriate HTTP request methods to interact with the server according to requirements, so as to realize different operations and functions.
POST request
POST request is one of the request methods used in HTTP protocol. It is used to submit data to a server for processing, storage, or other manipulation.
When using a POST request, the data is included in the request's message body, rather than appended to the URL's query string like in a GET request. This means that the data of the POST request will not be displayed directly in the URL and will not be visible to the user.
POST requests are typically used in the following situations:
Create resource: When you need to create a new resource on the server, you can use POST request. For example, submitting a form to create a new user or publishing a blog post.
Updating resources: When you need to update an existing resource on the server, you can use a POST request. For example, editing user profiles or modifying article content.
Deleting resources: When you need to delete a resource from the server, you can use a POST request. For example, deleting a user account or deleting a file.
Processing form data: When you need to submit form data to the server for processing, you can use POST request. Form data can contain various fields and values, such as a user registration form or a search form.
The data of the POST request will be wrapped in the request body and can be transmitted using various encoding methods, such as application/x-www-form-urlencoded, multipart/form-data, etc.
In web development, the server side needs to process POST requests accordingly, and parse the data in the request body to perform corresponding operations. In this way, operations such as data processing, verification, and persistence can be performed on the server, thereby realizing interaction with the client and data transmission.
Implement http service in Qt to receive POST request
To receive HTTP POST protocol data in Qt, you can use Qt's network module and HTTP class to handle the request. Here is a simple example showing how to use Qt to receive data from an HTTP POST request:
#include <QtNetwork>
#include <QTcpServer>
#include <QTcpSocket>
#include <QTextStream>
class HttpServer : public QTcpServer
{
Q_OBJECT
public:
explicit HttpServer(QObject *parent = nullptr) : QTcpServer(parent) {}
protected:
void incomingConnection(qintptr socketDescriptor) override
{
QTcpSocket *socket = new QTcpSocket(this);
socket->setSocketDescriptor(socketDescriptor);
connect(socket, &QTcpSocket::readyRead, this, &HttpServer::socketReadyRead);
connect(socket, &QTcpSocket::disconnected, this, &HttpServer::socketDisconnected);
}
private slots:
void socketReadyRead()
{
QTcpSocket *socket = qobject_cast<QTcpSocket *>(sender());
if (socket)
{
// Read the request from the socket
QByteArray requestData = socket->readAll();
// Parse the request
// In this example, we assume the request is in plain text format
QString request = QString::fromUtf8(requestData);
// Check if it's a POST request
if (request.startsWith("POST"))
{
// Extract the POST data
QString postData = request.split("\r\n\r\n").last();
// Process the POST data
processPostData(postData);
// Send a response back to the client
QString response = "HTTP/1.1 200 OK\r\n"
"Content-Type: text/plain\r\n"
"\r\n"
"POST data received!";
socket->write(response.toUtf8());
socket->flush();
socket->waitForBytesWritten();
}
socket->close();
}
}
void socketDisconnected()
{
QTcpSocket *socket = qobject_cast<QTcpSocket *>(sender());
if (socket)
{
socket->deleteLater();
}
}
private:
void processPostData(const QString &postData)
{
// Process the POST data here
qDebug() << "POST data received:" << postData;
}
};
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
HttpServer server;
if (!server.listen(QHostAddress::Any, 8080))
{
qDebug() << "Failed to start server.";
return 1;
}
qDebug() << "Server started on port 8080.";
return app.exec();
}
#include "main.moc"
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- twenty one.
- twenty two.
- twenty three.
- twenty four.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
- 63.
- 64.
- 65.
- 66.
- 67.
- 68.
- 69.
- 70.
- 71.
- 72.
- 73.
- 74.
- 75.
- 76.
- 77.
- 78.
- 79.
- 80.
- 81.
- 82.
- 83.
- 84.
- 85.
- 86.
- 87.
- 88.
- 89.
- 90.
- 91.
- 92.
In this example, we create a HttpServer class inherited from QTcpServer to handle HTTP requests. When a new connection request arrives, the incomingConnection function will be called, and a QTcpSocket will be created in this function to handle the connection. Then, connect the readyRead and disconnected signals of the socket to the corresponding slot functions.
In the socketReadyRead slot function, read the request and process it. If the request starts with "POST", we extract the POST data and call the processPostData function to process the data. You can process POST data in the processPostData function.
Finally, we send a simple response to the client and close the connection.
In the main function, we create an HttpServer instance and call the listen function to start listening for connections. If the listening fails, an error message will be output.
This is a simple example that demonstrates how to use Qt to receive data from an HTTP POST request. You can extend and modify it according to your specific needs, such as adding functions such as routing processing, verification and parsing of POST data.